How Observables work in KnockoutJs
This content is basically a translation of the original materials. The intention is to learn about KnockoutJs for Magento 2 and create content in Portuguese about KnockouJs.
Documentation
- KnockoutJs: Observables
- KnockoutJs: Observables Arrays
- KnockoutJs: Computed Observables
- KnockoutJs: Writable Computed Observables
- KnockoutJs: Pure Computed Observables
- KnockoutJs: Computed Observables
Observables
KnockoutJs introduces the concept of observables, which are properties that can be monitored and automatically updated when their values change. This functionality allows the user interface to dynamically react to changes in Model.
dataTo create an observable in KnockoutJs, you can use the ko.observable() function and assign an initial value to it. To access the current value of an observable, you can treat it as a function. To just observe something without an initial value, just call the Observable property without parameters.
let myObservable = ko.observable('Inicial'); console.log(myObservable()); // Irá imprimir 'Inicial' myObservable('Novo valor'); console.log(myObservable()); // Irá imprimir 'Novo valor'
- ko.isObservable: returns true for observables, observables arrays and all computed observables;
- ko.isWritableObservable: returns true for observables, observable arrays and writable computed observables.
Subscriptions
The subscriptions in observables are mechanisms that allow you to be notified whenever the value of an observable changes. They are essential for tracking changes in observables and reacting to these changes, updating the user interface or taking other actions when necessary.
The subscribe() method ***receives a *callback function that will be executed whenever the value of the observable is modified. The callback function receives as an argument the new value of the observable. This function accepts three parameters: callback is the function that is called whenever the notification happens, target (optional) defines the value of this in the function callback and event (optional; default is change) is the name of the event to receive notification.
let myObservable = ko.observable('Inicial'); console.log(myObservable()); // Irá imprimir 'Inicial' myObservable('Novo valor'); console.log(myObservable()); // Irá imprimir 'Novo valor'
- change: This is the default event that triggers the subscription whenever the value of the observable changes. It is the most common event and is used when no other event is explicitly specified;
- beforeChange: This event is fired before the value of the observable is changed. The callback function will receive two arguments: the current value of the observable and the proposed (new) value that will be assigned to the observable. This allows you to perform actions based on the current value before it is changed;
- afterChange: This event is triggered after the value of the observable is changed. The callback function will receive two arguments: the previous value of the observable (before the change) and the new value that was assigned to the observable. It's useful when you need to react to a specific change after it has occurred.
- arrayChange: This event is specific to Observables Arrays. It is triggered when there is a change to an observable array, such as adding, removing, or replacing items in the array. The callback function takes four arguments: the affected items (added, deleted, status and index).
Another important point is that it is possible to store the subscription in a variable and, if necessary, cancel the subscription using the dispose() method. This is useful when you want to temporarily or permanently disable UI updating in response to changes in observables.
let myObservable = ko.observable(0); // Criando uma subscription no observable myObservable.subscribe(function (newValue) { console.log('Novo valor do observable:', newValue); }, scope, event);
Methods for determining types of observables
- isObservable(): This method is used to check if a value is an observable. It returns true if the value is a observable (observable, observableArray, computed or writable computed), and false otherwise.
- isWritableObservable(): This method checks if a value is a writable observable (writable observable). It returns true if the value is a writable observable and false otherwise;
- isComputed(): This method is used to check if a value is a Computed Observable. It returns true if the value is a Computed Observable and false otherwise;
- isPureComputed(): This method checks if a value is a Pure Computed Observable. A Pure Computed Observable is one that depends only on other pure observables and has no internal recording logic. It returns true if the value is a Pure Computed Observable and false otherwise.
Observable Arrays
Observables Arrays are an extension of observables and are used to deal with lists of data that need to be observable. Unlike a standard JavaScript array, an Observable Array allows you to automatically track changes to list data and update the user interface reactively.
let myObservable = ko.observable('Inicial'); console.log(myObservable()); // Irá imprimir 'Inicial' myObservable('Novo valor'); console.log(myObservable()); // Irá imprimir 'Novo valor'
Observables Arrays have specific methods that allow you to add, remove and manipulate items reactively. Some of these methods are:
- indexOf(value): Returns the index of the first item of the array that is equal to its parameter, or the value -1 if no matching value is found.
- push(item): Adds a new item to the end of the array;
- pop(): Removes and returns the last item from the array;
- shift(): Removes and returns the first item from the array;
- unshift(item): Adds a new item to the beginning of the array;
- remove(item): Removes a specific item from the array;
- removeAll([parameter]): Removes all items from the array, and can receive a parameter in the form of array that will remove the items within the passed parameter(s);
- replace(oldItem, newItem): Replaces the item passed in the first parameter with the second parameter;
- reverse(): Changes the order of items in the original array and updates the user interface to reflect the new order;
- reversed(): Returns a reversed copy of the array;
- splice(index, count, items): Allows you to add or remove items in a specific position in the array;
- slice(): Returns a copy of a subset of the array, starting at the start index and going to the end-1 index. The start and end values are optional, and if not provided;
- sort(): Determines the order of items. If the function is not provided, the method sorts the items in ascending alphabetical order (for strings) or in ascending numerical order (for numbers);
- sorted(): Returns a copy of the sorted array. It is preferable to the sort() method if you need to not change the original observable array, but need to display it in a specific order;
For functions that modify the contents of the array, such as push and splice, the KO methods automatically trigger the dependency tracking mechanism so that all registered listeners are notified of the change and your interface is updated automatically, which means there is a significant difference between using KO methods (observableArray.push(...), etc) and native JavaScript array methods (observableArray() .push(...)), since the latter do not send any notification to array subscribers that their content has changed.
While it is possible to use subscribe and access an observableArray like any other observable, KnockoutJs also provides a super fast method to find out how an array observable did changed (which items were just added, deleted, or moved). You can subscribe to array changes as follows:
let myObservable = ko.observable('Inicial'); console.log(myObservable()); // Irá imprimir 'Inicial' myObservable('Novo valor'); console.log(myObservable()); // Irá imprimir 'Novo valor'
Computed Observables
Computed Observables are functions that depend on one or more observables and will be automatically updated whenever any of these dependencies change. The function will be called once each time any of its dependencies change, and any value it returns will be passed to the observables, such as UI elements or other computed observables .
The main difference between Computed Observables and Observables is that Computed Observables do not directly store a value; instead, they rely on other observables to calculate their value. This means that the value of a Computed Observable is always automatically updated when any of the observables that it depends on are modified.
let myObservable = ko.observable(0); // Criando uma subscription no observable myObservable.subscribe(function (newValue) { console.log('Novo valor do observable:', newValue); }, scope, event);
Methods of a Computed Observable
- dispose(): This method is used to dispose (clean up) a Computed Observable when it is no longer needed. It removes all subscriptions and dependencies associated with Computed Observable;
- extend(): This method allows you to add custom extenders to a Computed Observable. Extenders are functions that can modify the behavior of Computed Observable;
- getDependenciesCount(): This method returns the number of observables dependent on the Computed Observable;
- getDependencies(): This method returns an array containing the observables that are dependencies of the Computed Observable;
- getSubscriptionsCount(): This method returns the number of current subscriptions from the Computed Observable;
- isActive(): This method returns a Boolean value that indicates whether the Computed Observable is currently active (a Computed Observable is active if it is in the process of being evaluated due to a change in its dependencies );
- peek(): This method is similar to the parenthesis operator () used to access the current value of a Computed Observable. However, the peek method does not create a dependency between the Computed Observable and the place where it is called;
- subscribe(): This method allows you to subscribe to receive notifications whenever the value of Computed Observable changes.
this
The second parameter to ko.computed sets the value of this when evaluating the calculated observable. Without passing it, it would not be possible to reference this.firstName() or this.lastName().
There is a convention that avoids the need to track this completely: if the constructor of your viewmodel copies a reference to this into a different variable (traditionally called self), you can use self throughout your viewmodel and don't have to worry about it being redefined to refer to something else.
let myObservable = ko.observable('Inicial'); console.log(myObservable()); // Irá imprimir 'Inicial' myObservable('Novo valor'); console.log(myObservable()); // Irá imprimir 'Novo valor'
Because self is captured at function closure, it remains available and consistent in any nested function, such as the evaluator of the computed observable. This convention is even more useful when it comes to event handlers.
Pure computed observables
If a computed observable simply calculates and returns a value based on some observable dependencies, it is better to declare it as ko.pureComputed instead of ko.computed.
let myObservable = ko.observable(0); // Criando uma subscription no observable myObservable.subscribe(function (newValue) { console.log('Novo valor do observable:', newValue); }, scope, event);
When a computed observable is declared as pure, its evaluator does not directly modify other objects or state, KnockoutJs can more efficiently manage its re-evaluation and memory usage. KnockoutJs will automatically suspend or release it if no other code has an active dependency on it.
Writable Computed Observables
Writable Computed Observables are an extension of Computed Observables that allow the creation of computed observables that can be updated both through reading and writing . Unlike conventional Computed Observables, which only calculate their value based on other observables and do not directly store a value, Writable Computed Observables can store a value and also provide a function to update this value when necessary.
To create a Writable Computed Observable, it is necessary to use the ko.computed function with a configuration object that contains two main properties: read and write. The read property contains the calculation function to determine the value of the observable, while the write property contains the function that is called when you want to update the value of the observable.
The above is the detailed content of How Observables work in KnockoutJs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


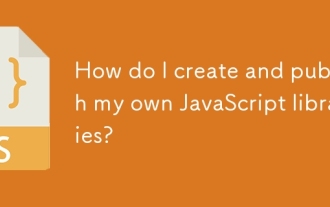
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
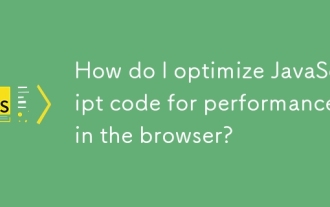
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
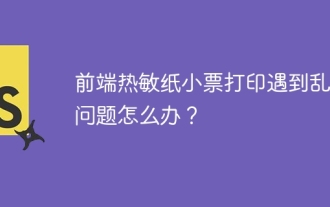
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
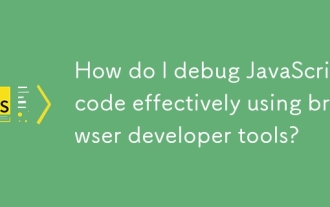
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
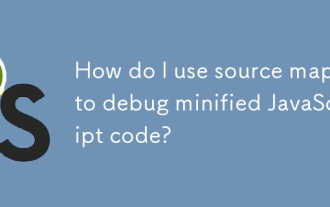
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
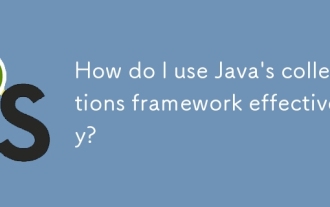
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
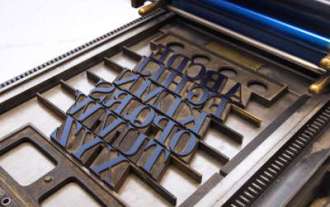
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
