


What\'s the difference between ranging over strings and rune slices in Go?
The Distinction Between Ranging Over Strings and Rune Slices
In Go, iterating using range over strings and rune slices might seem identical at first glance, as both yield the Unicode code points of the respective data structure. However, there's a crucial difference that becomes apparent when dealing with multibyte characters.
Ranging Over Strings
When you range over a string directly, like in the following code:
<code class="go">for _, s := range str { fmt.Printf("type of v: %s, value: %v, string v: %s \n", reflect.TypeOf(s), s, string(s)) }</code>
You're actually iterating over a sequence of bytes. As Go strings are essentially byte arrays, each iteration yields a byte from the string. This granularity may not pose an issue for strings primarily containing ASCII characters. However, for Unicode strings containing multibyte characters, byte-wise iteration could lead to unexpected results.
Ranging Over Rune Slices
In contrast, ranging over a rune slice, created by explicitly converting a string to a slice of runes like:
<code class="go">for _, s := range []rune(str) { fmt.Printf("type : %s, value: %v ,string : %s\n", reflect.TypeOf(s), s, string(s)) }</code>
Provides you with an iteration over code points. Unlike strings, rune slices are sequences of Unicode characters, making them more suitable for operating on textual data at the character level.
Implications for Indexing
The choice between ranging over strings and rune slices becomes even more critical when using indexing. Indexing a string will give you the byte position of a character, while indexing a rune slice will provide the index of the character within the sequence of code points.
For example, if you have a string with a multibyte character at index 1, indexing it as a rune slice would provide the index of that character, which may be different from the byte index.
Conclusion
In Go, ranging over strings and rune slices serves different purposes. Ranging over strings gives you bytes, while ranging over rune slices provides character-level iteration. The decision between the two depends on whether you need to work with bytes or characters, and whether indexing is a factor. For general-purpose text manipulation, rune slices are the preferred choice, ensuring consistent character-based operations regardless of character encoding.
The above is the detailed content of What\'s the difference between ranging over strings and rune slices in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










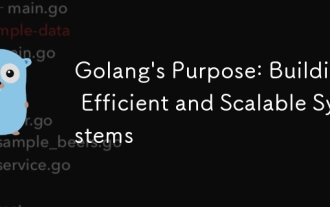
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
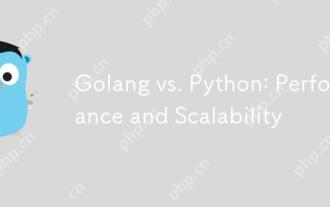
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
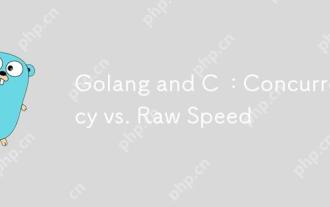
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
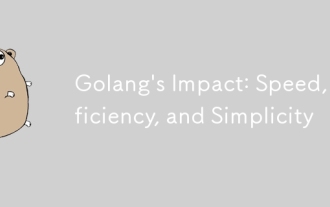
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
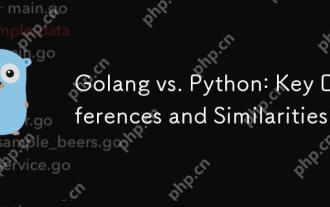
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
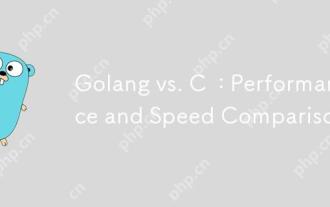
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
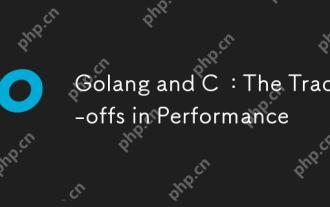
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
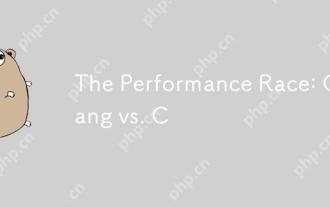
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
