


How can I efficiently search for and list files within subfolders using Python?
Recursive Subfolder Search and File Listing
It is common to encounter scenarios where you need to traverse through multiple subfolders and extract specific files based on their file extension. One approach to tackle this is by leveraging Python's built-in os.walk function, which enables recursive exploration of directories and their contents.
However, as encountered in this question, the problematic behavior arises when attempting to concatenate the files' paths. The subFolder variable returned by os.walk represents a list of subfolders rather than the specific folder where each file resides.
To remedy this issue, the correct approach is to utilize the dirpath (or root in the given code), which represents the current directory for the iteration. The dirpath value should be concatenated with the file name to construct the complete file path.
Furthermore, it is important to consider additional factors such as pruning certain folders from the recursion process, which can be achieved by examining the dn (dirname) list. To avoid relying on string manipulation for file extension checks, you can leverage the os.path.splitext function.
Here is an optimized version of the code that incorporates these enhancements:
<code class="python">import os result = [ os.path.join(dp, f) for dp, dn, filenames in os.walk(PATH) for f in filenames if os.path.splitext(f)[1] == ".txt" ]</code>
As an alternative, glob is another powerful tool that can be employed to select files based on their extensions. Here's an example using glob:
<code class="python">import os from glob import glob result = [ y for x in os.walk(PATH) for y in glob(os.path.join(x[0], "*.txt")) ]</code>
For Python 3.4 , Pathlib provides an intuitive approach for this task:
<code class="python">from pathlib import Path result = list(Path(".").rglob("*.[tT][xX][tT]"))</code>
The above is the detailed content of How can I efficiently search for and list files within subfolders using Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










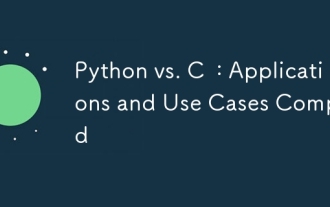
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
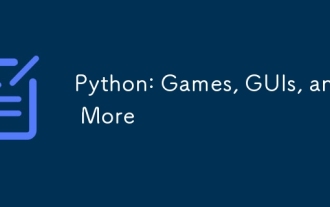
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
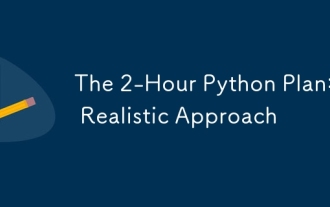
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
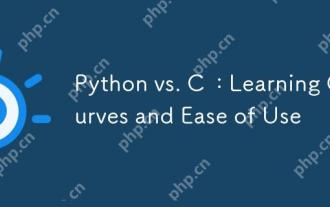
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
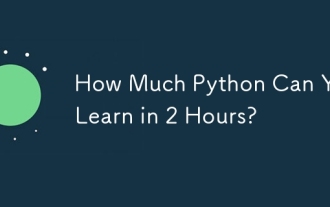
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
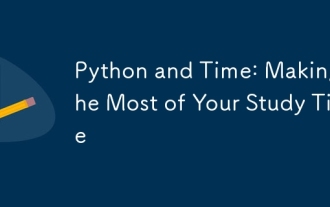
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
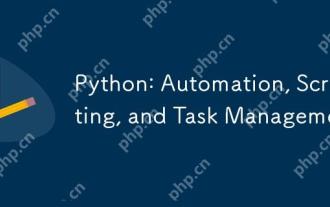
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
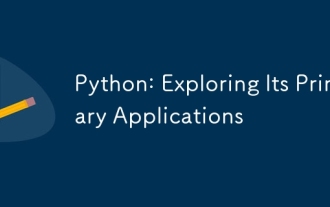
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
