What is decltype in C and how does it work?
Understanding Decltype for Beginners
Decltype is a C keyword that allows you to deduce the type of an entity based on an expression. It is particularly useful in writing generic library code where the actual type of an object may not be known in advance.
Syntax of Decltype
The syntax of decltype is:
decltype(expression)
How Decltype Works
Decltype takes an expression as its argument and returns a type that corresponds to the type of the expression. Here are a few scenarios explaining how it works:
- If the expression is an unparenthesized variable or class member access, decltype returns the type of that entity.
- If the expression is an xvalue (an expression that can be moved from), decltype returns a reference to that type.
- If the expression is an lvalue (an expression that cannot be moved from), decltype returns a reference to that type.
- If none of the above conditions match, decltype returns the type of the expression.
Example Code Explanation
Consider the following code snippet:
int a = 3, b = 4; decltype(a) c = a; decltype((b)) d = a; ++c; ++d;
- Line 1: decltype(a) specifies that c is of the same type as a, which is an int.
- Line 2: decltype((b)) specifies that d is a reference to the same type as b, which is an int. However, since a is an lvalue, d is a reference to an int.
- Line 3 and 4: The increment operations c and d add 1 to the respective variables. c is directly incremented since it is of type int, while d is dereferenced before being incremented.
Applications of Decltype
Decltype is commonly used in generic programming, where the type of an object is not known in advance:
- To define template functions or classes that can work with different types.
- To perform constexpr type computations, such as determining the size of a data structure or the return type of a function.
- To avoid unnecessary copying or moving of objects, by ensuring that the correct type of reference is returned.
Difference Between Decltype and Auto
While both decltype and auto can be used for type deduction, they have different applications:
- Decltype deduces the type based on an expression, regardless of the scope in which the expression is used.
- Auto deduces the type within the scope where the variable is declared, based on its initializer.
Conclusion
Decltype provides a powerful mechanism for working with types in C , allowing programmers to write generic and efficient code. While it may not be needed in everyday programming for beginners, it is a valuable tool for advanced developers and library designers.
The above is the detailed content of What is decltype in C and how does it work?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










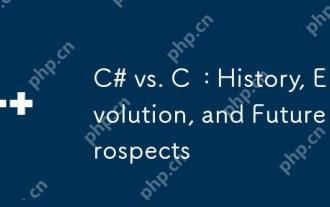
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
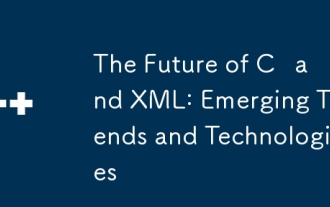
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
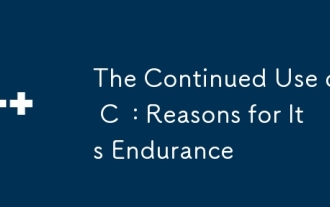
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
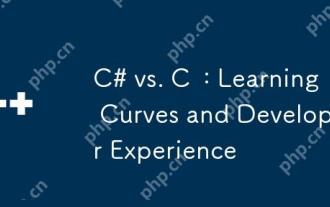
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
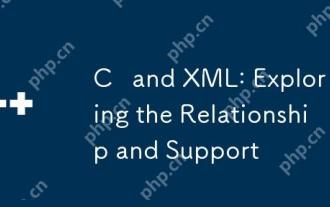
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
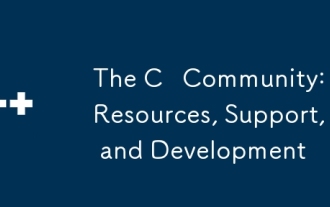
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
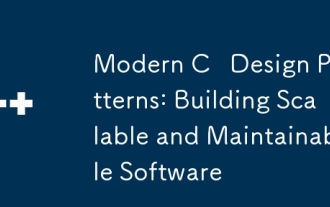
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
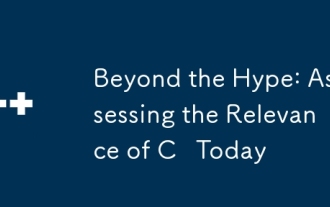
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
