How to Decode Compressed Images Using a Custom Input Stream in C ?
How to Write a Custom Input Stream in C
Introduction
C stream input/output (IO) operations are commonly performed using the iostream library. While this library provides a solid foundation for most IO tasks, there are scenarios where you may need to implement custom input streams to handle data in non-standard formats.
Overview of Custom Input Streams
Custom input streams are defined by extending the std::streambuf class and overriding its virtual methods, such as underflow() for reading and overflow() and sync() for writing. By overriding these functions, you can control the way the stream interacts with the underlying data source or destination.
Example: Vertical XOR Image Decoding
Let's consider a specific example of implementing a custom input stream for decoding images that have been compressed using vertical XOR encoding.
<code class="cpp">class vxor_streambuf : public std::streambuf { public: // Constructor takes the original buffer and image width vxor_streambuf(std::streambuf *buffer, int width) : buffer(buffer), size(width / 2) { // Allocate memory for previous and current lines previous_line = new char[size]; memset(previous_line, 0, size); current_line = new char[size]; // Initialize streambuf member variables setg(0, 0, 0); setp(current_line, current_line + size); } // Destructor releases memory ~vxor_streambuf() { sync(); delete[] previous_line; delete[] current_line; } // Underflow() performs XOR decoding for reading std::streambuf::int_type underflow() { // Read line from original buffer streamsize read = buffer->sgetn(current_line, size); if (!read) return traits_type::eof(); // Perform vertical XOR decoding for (int i = 0; i < size; i += 1) { current_line[i] ^= previous_line[i]; previous_line[i] = current_line[i]; } // Update streambuf member variables setg(current_line, current_line, current_line + read); return traits_type::to_int_type(*gptr()); } // Overflow() performs XOR encoding for writing std::streambuf::int_type overflow(std::streambuf::int_type value) { int write = pptr() - pbase(); if (write) { // Perform vertical XOR encoding for (int i = 0; i < size; i += 1) { char tmp = current_line[i]; current_line[i] ^= previous_line[i]; previous_line[i] = tmp; } // Write line to original buffer streamsize written = buffer->sputn(current_line, write); if (written != write) return traits_type::eof(); } // Update streambuf member variables setp(current_line, current_line + size); if (!traits_type::eq_int_type(value, traits_type::eof())) sputc(value); return traits_type::not_eof(value); }; // Sync() flushes any pending data virtual int sync() { streambuf::int_type result = this->overflow(traits_type::eof()); buffer->pubsync(); return traits_type::eq_int_type(result, traits_type::eof()) ? -1 : 0; } private: streambuf *buffer; int size; char *previous_line; char *current_line; };</code>
Usage
<code class="cpp">ifstream infile("encoded_image.vxor"); vxor_istream in(infile, 288); // Create a new vxor_istream char data[144 * 128]; in.read(data, 144 * 128); // Read encoded data</code>
Additional Considerations
- Performance: Custom streams can impact performance since they introduce an additional layer of indirection.
- Robustness: Ensure that your custom stream handles errors and exceptions gracefully to maintain data integrity.
- Documentation: Provide clear documentation for your custom streams to help others understand their usage.
By following these guidelines and understanding the intricacies of streambuf manipulation, you can effectively implement custom input streams for various scenarios where standard IO operations fall short.
The above is the detailed content of How to Decode Compressed Images Using a Custom Input Stream in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
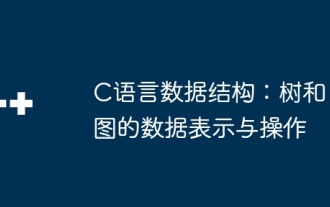
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
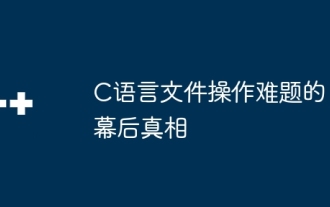
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
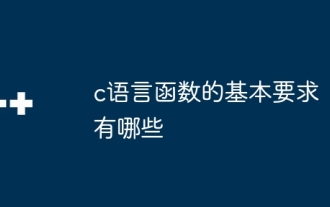
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
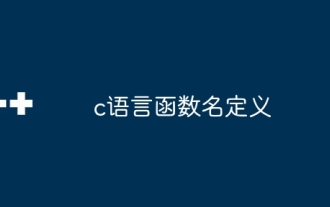
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
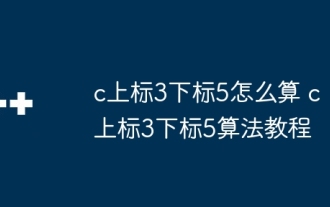
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
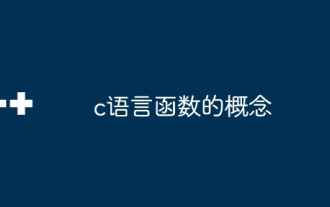
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
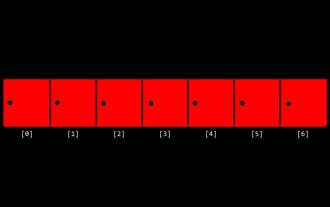
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
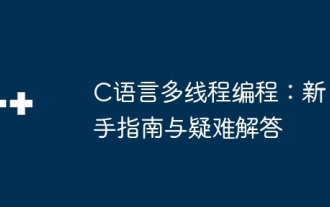
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
