


Functions as Struct Fields vs. Struct Methods: When to Use Which in Go?
Functions as Struct Fields vs. Struct Methods
In Go, functions can be embedded as fields within structs or defined as methods of those structs. Understanding the distinctions between these approaches can optimize your code design.
Fields of Function Type
Fields of function type are not true methods attached to the struct type. They store a reference to a function rather than being part of the struct's method set.
True Methods
True methods, declared with the struct type as the receiver, are integral to the struct's method set. They allow for implementing interfaces and operating on concrete types. Once defined, methods cannot be changed at runtime.
When to Use Fields of Function Type
- For callbacks, such as event handlers or network request handlers.
- To mimic virtual methods by assigning a function to a field and reassigning it at runtime.
When to Use True Methods
- When implementing interfaces
- When working with concrete types and requiring a consistent method set
- When security is a concern, as methods are immutable and field access can potentially be tampered with
Example
<code class="go">type Foo struct { Bar func() } func main() { f := Foo{ Bar: func() { fmt.Println("initial") }, } f.Bar() f.Bar = func() { fmt.Println("changed") } f.Bar() }</code>
Output:
initial changed
In this example, a function is embedded as a field in the Foo struct. By reassigning the field at runtime, we can change the behavior of the Bar method, demonstrating the flexibility of fields of function type.
The above is the detailed content of Functions as Struct Fields vs. Struct Methods: When to Use Which in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










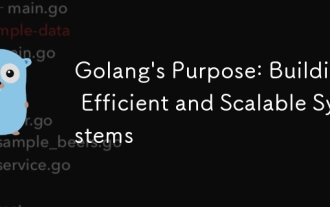
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
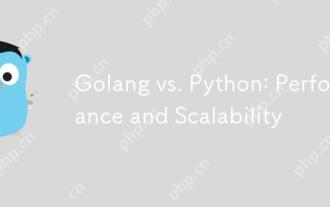
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
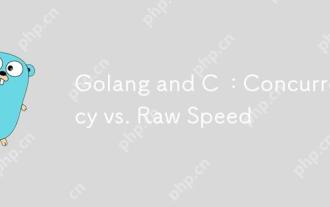
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
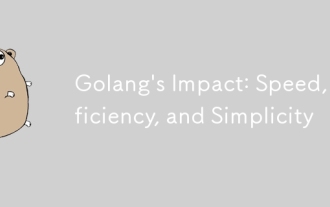
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
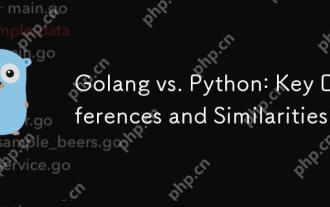
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
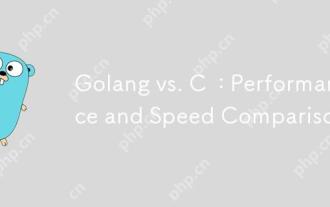
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
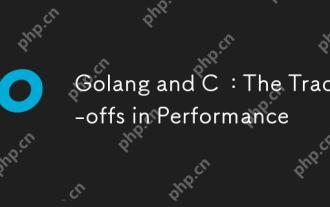
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
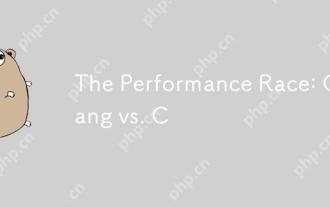
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
