


Why does assigning a value to a complex variable cause an infinite loop in a C program, even though it appears to be a seemingly innocuous action?
C Compilation Enigma with Unpredictable Loop Behavior
The following code presents an intriguing compilation issue:
<code class="c++">#include <iostream> #include <complex> using namespace std; int main() { complex<int> delta; complex<int> mc[4] = {0}; for(int di = 0; di < 4; di++, delta = mc[di]) { cout << di << endl; } return 0; }</code>
Contrary to the expected output of "0, 1, 2, 3" terminating, the code produces an endless repetition of "0, 1, 2, 3, 4, 5, ...". Upon investigation, it appears that the comparison di < 4 fails to work correctly, resulting in an infinite loop.
Disabling the seemingly innocuous assignment delta = mc[di] magically fixes the issue, generating the intended output. What is the problem that this simple action causes?
Delving into Undefined Behavior
The key to unraveling this mystery lies in understanding undefined behavior. The assignment delta = mc[di] triggers an access to an array out of bounds on the final iteration of the loop. While many compilers might assume no undefined behavior based on optimization strategies, this assumption is inherently flawed.
GCC, with optimizations turned on, can aggressively optimize the loop assuming the absence of undefined behavior. This optimization leads to an erroneous inference that di < 4 is always true, since an out-of-bounds array access would constitute undefined behavior.
As a result, the loop becomes an infinite one, as the condition di < 4 is always met. To prevent this erroneous optimization, the -fno-aggressive-loop-optimizations flag can be added to GCC.
Unveiling the Compiler's Inner Workings
A closer examination of the optimized code reveals that the di < 4 check is removed and replaced with an unconditional jmp instruction. This behavior aligns with the assumption of no undefined behavior, resulting in the infinite loop.
Contrary to this behavior, Clang with -fsanitize=undefined would catch this case. However, GCC with the same flag fails to raise a warning in this specific instance.
The Perils of Undefined Behavior
Undefined behavior, as defined by the C standard, allows for unpredictable results, including completely disregarding the situation. Undefined behavior should be avoided at all costs, as it can lead to both compiler inconsistencies and unexpected runtime behavior.
To avoid such pitfalls, programmers should strive for well-defined code that adheres to the intended semantics. Compilers should also implement appropriate defenses to alert developers to potential undefined behavior.
The above is the detailed content of Why does assigning a value to a complex variable cause an infinite loop in a C program, even though it appears to be a seemingly innocuous action?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










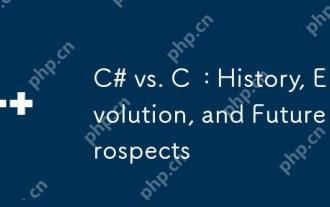
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
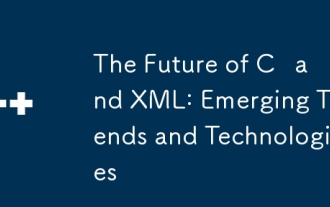
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
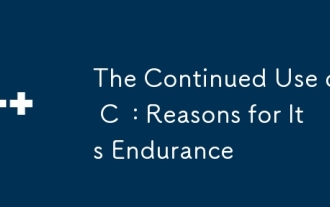
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
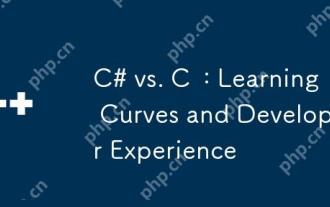
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
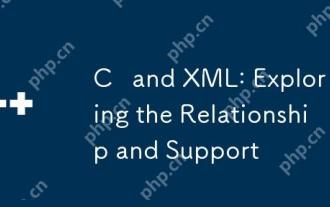
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
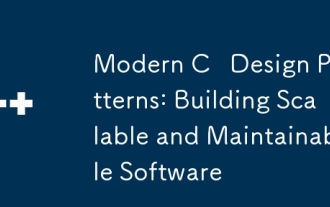
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
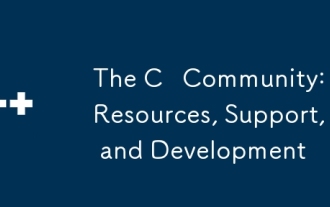
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
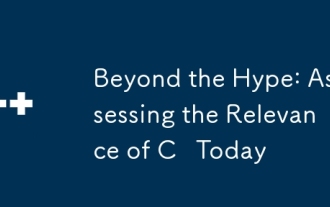
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
