


How to Efficiently Convert UTF8 Strings to Byte Arrays for JSON Unmarshaling in Go?
Converting UTF8 Strings to Byte Arrays for JSON Unmarshaling
When unmarshaling a JSON string using the Unmarshal function, a parameter representing a byte array is required. This article provides an efficient method to convert a UTF8 string into a compatible byte array.
Conversion Process
The Go language allows direct conversion from strings to byte arrays, as specified in the language specification. The following code demonstrates this conversion:
<code class="go">s := "some text" b := []byte(s) // b is of type []byte</code>
However, this conversion can be inefficient for large strings due to the copy operation it performs. An alternative approach is to utilize an io.Reader to avoid the copy overhead. This involves creating an io.Reader using strings.NewReader() and passing it to json.NewDecoder() instead:
<code class="go">s := `{"somekey":"somevalue"}` var result interface{} err := json.NewDecoder(strings.NewReader(s)).Decode(&result) fmt.Println(result, err)</code>
This method allows the program to access the JSON data without creating a copy of the input string.
Performance Considerations
The overhead associated with creating an io.Reader and using json.NewDecoder() should be considered for small JSON texts. In such cases, direct conversion via []byte(s) is still a viable option as it provides comparable performance.
Conclusion
Depending on the size and source of the JSON input, different methods can be utilized to convert UTF8 strings to byte arrays for unmarshaling. By leveraging the direct conversion or io.Reader approach, developers can effectively process JSON data in their Go applications.
The above is the detailed content of How to Efficiently Convert UTF8 Strings to Byte Arrays for JSON Unmarshaling in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










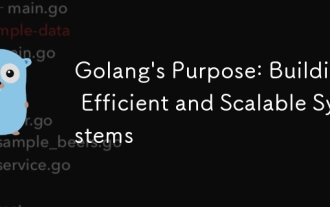
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
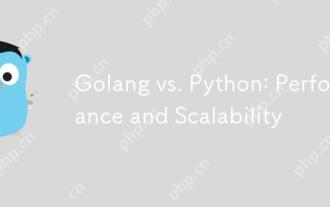
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
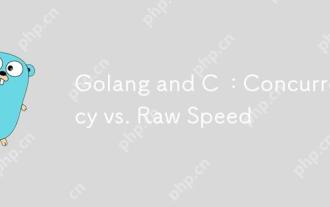
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
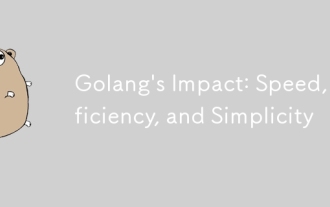
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
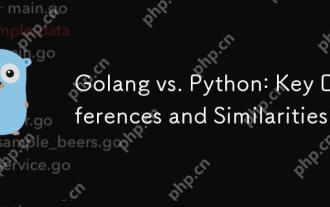
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
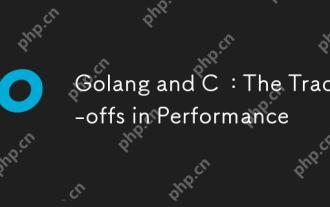
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
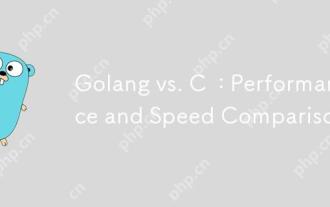
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
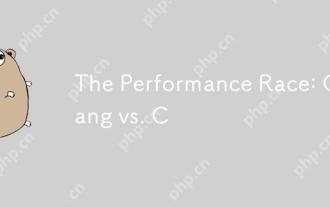
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
