


How to Handle Exceptions Effectively in Java and C#: Best Practices and When to Throw the Towel?
Exception Management Best Practices in Java and C
Exception handling is a crucial aspect of robust software development. It allows you to gracefully handle unexpected errors and prevent system crashes. However, there are certain best practices to follow to ensure effective exception management.
Catching Exceptions for Handling
- Is it okay to catch exceptions and not propagate them?
Generally, it's not advisable to simply catch exceptions and return error codes without propagating them. Exceptions provide valuable information about the root cause of the problem, which can aid in resolving the issue.
Exception Handling Best Practices
- Only catch what you can handle: Catch exceptions that your code can proactively address, such as recovering from transient network errors or gracefully handling deserialization exceptions.
- Avoid excessive try/catch blocks: Use try/catch blocks sparingly. Excessive try/catch statements can make code cluttered and difficult to maintain.
- Use specific exception types: Catch specific exception types instead of generic ones (e.g., IOException instead of Exception) to enhance error handling precision.
- Rethrow unhandled exceptions: In cases where you cannot handle an exception, rethrow it to allow higher-level code to handle it appropriately.
- Log and notify for unhandled exceptions: Include a top-level exception handler to log unhandled exceptions and notify the user (if necessary).
- Respect language idioms: Consider the error management constructs provided by the language (e.g., exceptions in Java and C#) and avoid introducing unnecessary complexity.
- Use exceptions for exceptional scenarios: Exceptions should be reserved for truly exceptional situations and not for normal flow control.
Conclusion
Effective exception management involves carefully handling exceptions that you can resolve, providing appropriate information for further investigation, and ensuring that the system gracefully recovers from unexpected failures. By following best practices, you can enhance code reliability and maintainability.
The above is the detailed content of How to Handle Exceptions Effectively in Java and C#: Best Practices and When to Throw the Towel?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










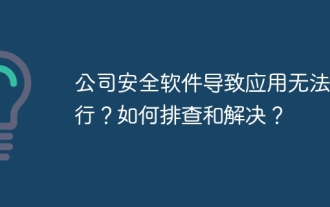
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
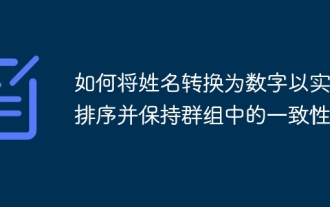
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
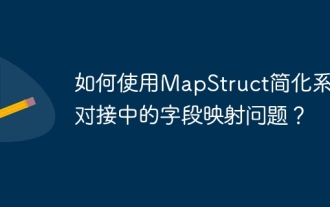
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
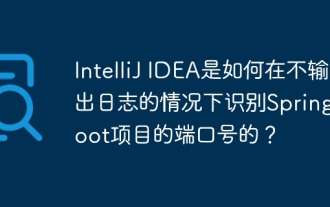
Start Spring using IntelliJIDEAUltimate version...
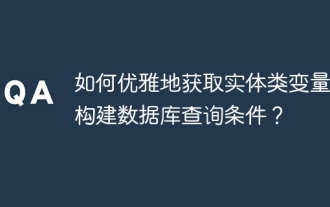
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
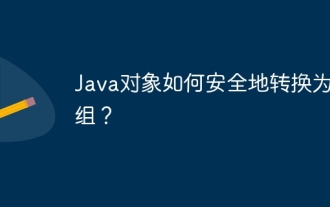
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
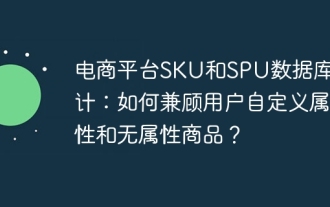
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
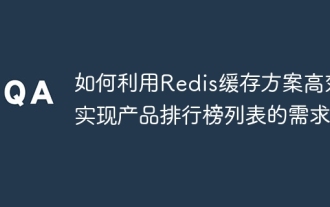
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
