Is My Port Open? A Java Implementation for Checking Port Availability.
Oct 30, 2024 am 05:37 AMSockets: Determining Port Availability in Java
In the realm of networking, it's often invaluable to determine the availability of specific ports on a machine. Java provides a versatile means to programmatically check this, ensuring optimal network communication.
Implementation
One effective Java implementation suggested by the Apache Camel project is as follows:
<code class="java">public static boolean available(int port) { if (port < MIN_PORT_NUMBER || port > MAX_PORT_NUMBER) { throw new IllegalArgumentException("Invalid start port: " + port); } ServerSocket ss = null; DatagramSocket ds = null; try { ss = new ServerSocket(port); ss.setReuseAddress(true); ds = new DatagramSocket(port); ds.setReuseAddress(true); return true; } catch (IOException e) { } finally { ... // Socket cleanup } return false; }</code>
This method ensures that the port is available not only for TCP but also for UDP by using both ServerSocket and DatagramSocket. By attempting to create and open sockets on the specified port, we can determine its availability.
Usage
To leverage this function, simply pass the port number in question. The code will return a boolean value, indicating whether the port is currently in use or available.
Conclusion
This Java implementation provides an efficient and reliable way to ascertain port availability on a given machine. By utilizing this method, developers can optimize their network applications to avoid conflicts and ensure seamless communication.
The above is the detailed content of Is My Port Open? A Java Implementation for Checking Port Availability.. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
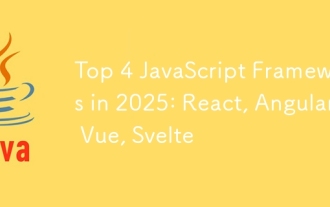
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
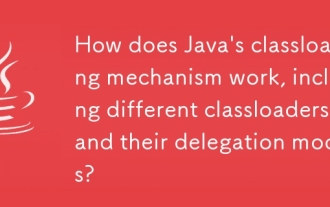
How does Java's classloading mechanism work, including different classloaders and their delegation models?
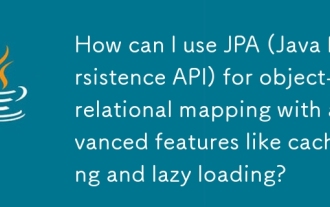
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
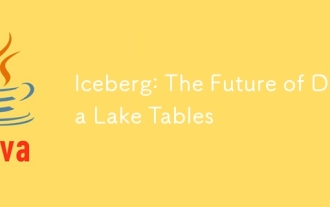
Iceberg: The Future of Data Lake Tables
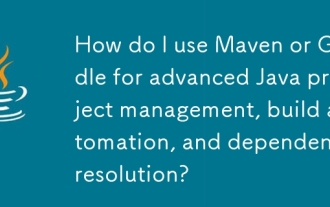
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
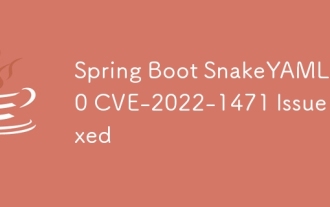
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
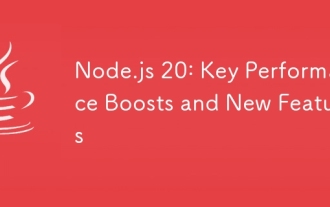
Node.js 20: Key Performance Boosts and New Features
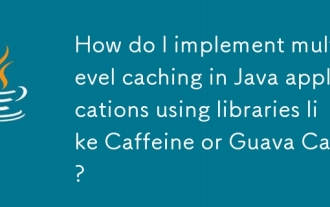
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
