How Does the Modulo Operator Work in Java?
Mod Operator in Java: Syntax and Semantics
In programming languages, the modulo operator (%) calculates the remainder of a division operation. In Java, the modulo operator is used for non-negative integers. However, it differs slightly in semantics from the pseudocode you provided.
Syntax for Modulo Operator:
The syntax for the modulo operator in Java is:
<code class="java">int result = number % divisor;</code>
Example:
The following code demonstrates the use of the modulo operator:
<code class="java">int number = 10; int divisor = 3; int remainder = number % divisor; System.out.println(remainder); // Output: 1</code>
The result of the modulo operator is 1, which is the remainder when 10 is divided by 3.
Alternative for Even/Odd Check:
In your pseudocode example, you used the modulo operator to check if a number is even or odd. However, Java uses the remainder operator %, not the modulo operator, for this purpose. The syntax for the remainder operator is the same as for the modulo operator.
<code class="java">if ((a % 2) == 0) { isEven = true; } else { isEven = false; }</code>
Simplified Version:
You can simplify the above code using a one-liner:
<code class="java">isEven = (a % 2) == 0;</code>
The above is the detailed content of How Does the Modulo Operator Work in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










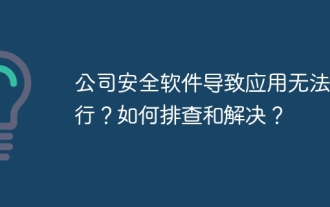
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
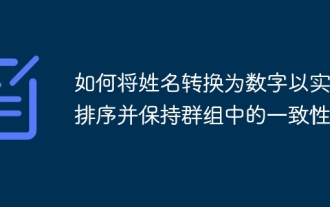
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
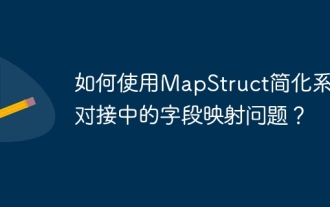
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
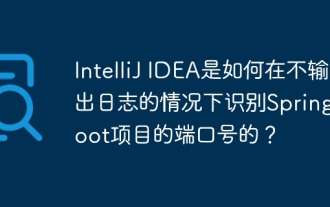
Start Spring using IntelliJIDEAUltimate version...
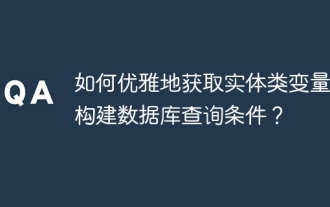
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
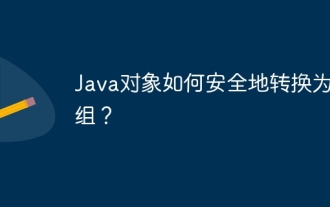
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
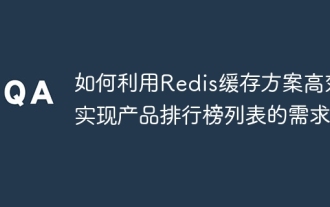
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
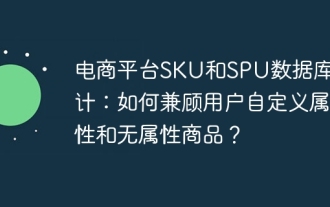
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
