How to Assert on a Log Message in JUnit?
How to Assert on a Log Message in JUnit
Introduction:
Testing the behavior of code that communicates with external systems often involves verifying the content and format of messages generated. In Java, logging is a common way to record such messages. This article explores how to perform unit testing on log entries using the JUnit framework.
JUnit Assertions on Log Messages:
The code snippet provided in the question aims to assert that a certain log entry with a specific level (e.g., INFO) and message ("x happened") was generated by the code under test. To achieve this, you can employ a custom logger or handler that intercepts and stores log events.
Custom Logger/Handler Approach:
This approach requires creating a specialized logger or handler that captures logging events. The log statements in the code under test are then directed to this custom logger, enabling the retrieval and inspection of logged messages. However, this approach can be complex to implement and requires modifying the code under test.
Log Collector Pattern:
A more convenient solution is to use a custom appender that records logging events in a collection or list. Here's how you can achieve this:
-
Create an Appender:
Implement a custom Appender subclass, overriding the append() method to store logging events in a data structure (e.g., a list). -
Add Appender to Logger:
Add an instance of your custom appender to the desired logger. This step can be performed within the test method or using annotations like @Before and @After. -
Logging Message Assertion:
After executing the code under test, retrieve the list of logged events from your appender. You can then use JUnit's assertion methods to verify the level, message, and other attributes of the log entry, as demonstrated in the provided code snippet.
Sample Implementation:
The following sample code illustrates the log collector pattern:
<code class="java">import org.apache.log4j.AppenderSkeleton; import org.apache.log4j.Level; import org.apache.log4j.Logger; import org.junit.Test; import java.util.ArrayList; import java.util.List; import static org.junit.Assert.assertThat; import static org.hamcrest.CoreMatchers.is; public class LogCollectorTest { @Test public void testLogMessage() { // Create a custom appender to collect logging events. final TestAppender appender = new TestAppender(); // Add the appender to the logger we wish to test. final Logger logger = Logger.getLogger(LogCollectorTest.class); logger.addAppender(appender); // Execute code under test. logger.info("Test"); // Retrieve the list of logged events. final List<LoggingEvent> logs = appender.getLog(); // Perform assertions on the first log entry. assertThat(logs.get(0).getLevel(), is(Level.INFO)); assertThat(logs.get(0).getMessage(), is("Test")); } private static class TestAppender extends AppenderSkeleton { private final List<LoggingEvent> log = new ArrayList<>(); @Override public boolean requiresLayout() { return false; } @Override protected void append(LoggingEvent loggingEvent) { log.add(loggingEvent); } @Override public void close() { } public List<LoggingEvent> getLog() { return new ArrayList<>(log); } } }</code>
Conclusion:
The log collector pattern provides a reusable and convenient way to assert on log messages in JUnit tests. By capturing logged events in a data structure, you can easily verify their content and format, ensuring the correctness of your code's interaction with logging systems.
The above is the detailed content of How to Assert on a Log Message in JUnit?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
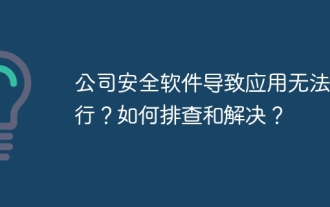
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
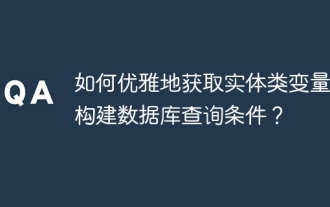
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
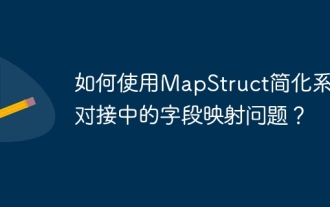
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
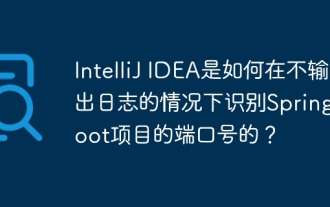
Start Spring using IntelliJIDEAUltimate version...
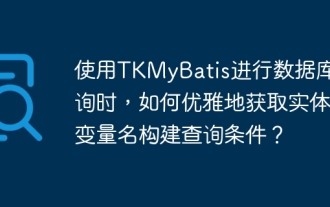
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
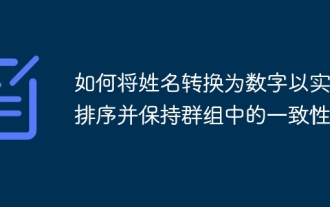
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
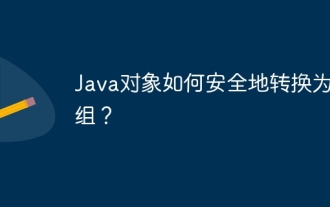
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
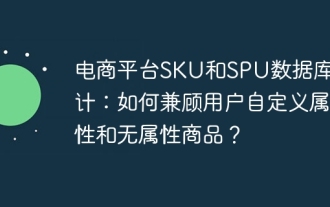
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
