


How to Convert Go []byte to Little/Big-Endian Signed Integer or Floating-Point Numbers?
How to Convert Go []byte to Little/Big-Endian Signed Integer or Float?
When dealing with binary data, it's crucial to understand the concept of endianness, which refers to the way multi-byte numeric values are stored in memory. Go's binary package provides convenient functions for converting between big-endian and little-endian unsigned integer types like Uint16 and Uint32. However, it lacks direct support for signed integers and floating-point numbers.
The key to overcoming this limitation is to approach the task in two steps:
- Assemble the numeric value in the desired byte order using BigEndian or LittleEndian.
- Interpret the resulting bytes as the appropriate signed integer or float type.
Converting to Signed Integers
To interpret a uint16 as a signed int16, for instance, simply use a type conversion, as they share the same memory layout:
<code class="go">a := binary.LittleEndian.Uint16(sampleA) a2 := int16(a)</code>
Similar principles apply for larger signed integer types like int64.
Converting to Floating-Point Numbers
While converting unsigned integers to floating-point types cannot be done directly through a type conversion, Go's math package provides functions specifically designed for this purpose. These functions allow you to convert unsigned integers to floats and vice versa without altering the underlying memory representation:
<code class="go">a := binary.LittleEndian.Uint64(sampleA) a2 := math.Float64frombits(a) // To convert back to unsigned integer: a3 := math.Float64bits(a2)</code>
Alternative Using binary.Read() and Write()
Go's binary.Read and Write functions can be used to perform these conversions more efficiently by accessing the underlying memory representation directly. Check out the example below:
<code class="go">pi := float64(3.141592653589793) buf := new(bytes.Buffer) binary.Write(buf, binary.LittleEndian, &pi) var result float64 binary.Read(buf, binary.LittleEndian, &result) fmt.Println(result)</code>
The above is the detailed content of How to Convert Go []byte to Little/Big-Endian Signed Integer or Floating-Point Numbers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










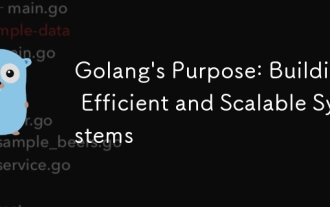
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
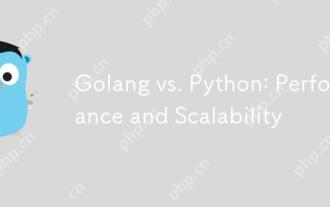
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
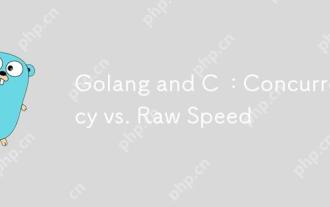
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
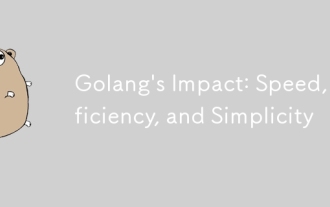
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
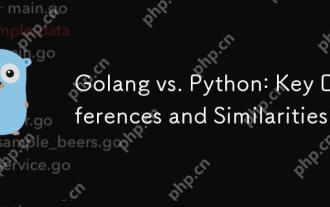
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
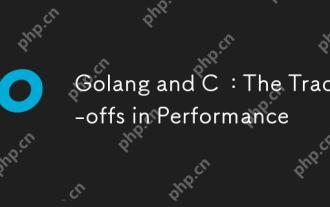
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
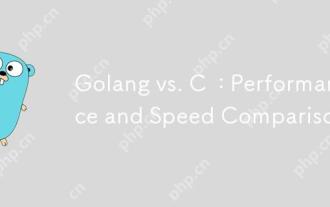
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
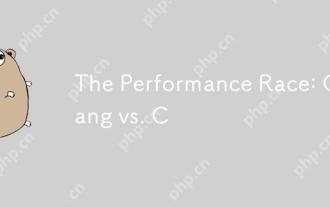
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
