


How to Resolve the \'Cannot Bind Non-Const Lvalue Reference\' Error in C Constructors?
Handling "Cannot Bind Non-Const Lvalue Reference" Error in C Constructor
When attempting to initialize a private object within a constructor, encountering the "cannot bind non-const lvalue reference of type 'int&' to an rvalue of type 'int'" error can be frustrating. This error occurs when an lvalue reference (an alias to a named variable) is expected but the argument passed is an rvalue (a temporary or literal value).
In the given code, the error arises because the Foo class constructor takes a non-const reference parameter (int &x), which must refer to an lvalue. However, the genValue() function returns an rvalue, as it creates a temporary int variable that is destroyed once the function returns.
To resolve this error, we must avoid binding the lvalue reference to an rvalue. Here's a breakdown of some potential solutions:
Pass by Value
The most straightforward solution is to pass the value returned by genValue() by value to the Foo constructor. This means changing the constructor in Foo class from Foo(int &x) to Foo(int x).
Create a Temporary Variable
Alternatively, you can create a temporary int variable inside the constructor of Bar, assign the result of genValue() to it, and then pass the reference to that temporary variable to the Foo constructor. This approach ensures that an lvalue is available to bind to the non-const reference.
Use Smart Pointers
If you're willing to use smart pointers, you can create a unique_ptr to manage the lifetime of the Foo object. This allows you to pass the pointer to the Foo object to the Foo constructor by reference, as it is an lvalue.
By implementing one of these solutions, you can effectively handle the aforementioned error and correctly initialize the private Foo object in the constructor of Bar.
The above is the detailed content of How to Resolve the \'Cannot Bind Non-Const Lvalue Reference\' Error in C Constructors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










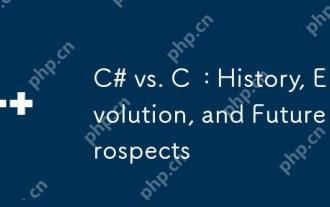
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
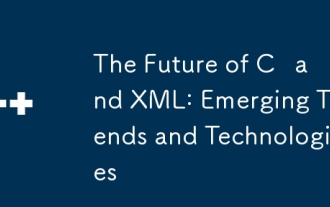
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
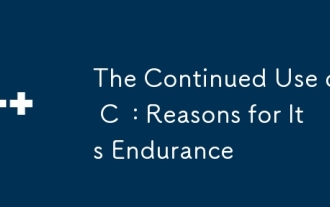
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
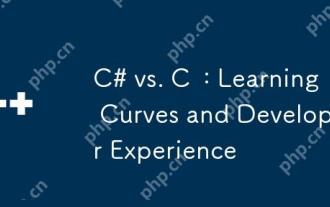
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
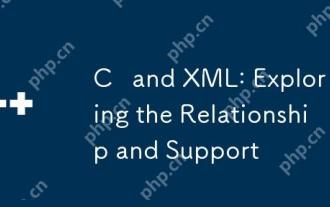
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
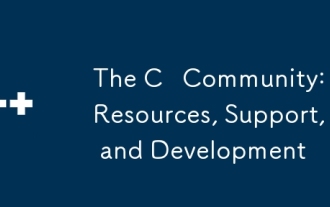
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
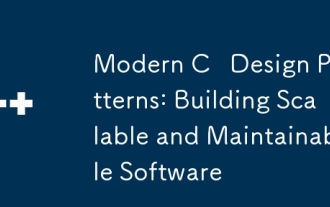
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
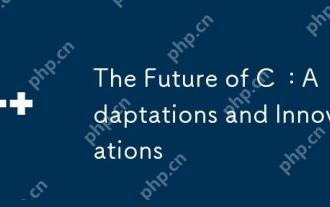
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
