


How to Pickle a Chorus of Objects: Saving and Loading Multiple Instances with Python\'s Pickle?
Pickle a Chorus of Objects: How to Save and Load Multiple Instances
Python's pickle module offers a convenient means of serializing objects to a file, enabling their persistence for later use. But what about scenarios where multiple objects require preservation? This article delves into the methods of handling such situations.
The Pickle Conundrum: A Tale of One or Many
As you've discovered, pickle excels in saving single objects. However, extending this functionality to multiple objects raises questions: Can they be saved collectively? Are there alternatives involving lists or other approaches?
Embracing the Power of Pickles: Collective Serialization
Rest assured, pickle's capabilities extend to preserving multiple objects within a single file. The key to this ensemble approach lies in a for loop that iterates over the objects, serializing each one using pickle.dump().
<code class="python">import pickle # Sample list of players players = [Player1, Player2, Player3] with open('players.pkl', 'wb') as f: for player in players: pickle.dump(player, f)</code>
Retrieving the Pickled Ensemble: Unveiling the Saved Melodies
Once the players have been pickled, retrieval is a simple reverse process. Using a for loop again, iterate over the pickle file and load each object with pickle.load().
<code class="python">import pickle with open('players.pkl', 'rb') as f: loaded_players = [] while True: try: loaded_players.append(pickle.load(f)) except EOFError: break</code>
Optimizing the Pickle Symphony: Two Additions
Beyond the fundamental approach, consider these enhancements:
- Avoid Explicit Length Storage: Use a generator to load objects continuously until the file's end is reached, significantly reducing memory consumption.
- Generator Benefits: Embracing a generator offers memory-friendly incremental loading, especially valuable for large datasets.
By incorporating these techniques, you'll master the art of saving and loading multiple objects with pickle, turning your software into a symphony of seamlessly persistent melodies.
The above is the detailed content of How to Pickle a Chorus of Objects: Saving and Loading Multiple Instances with Python\'s Pickle?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


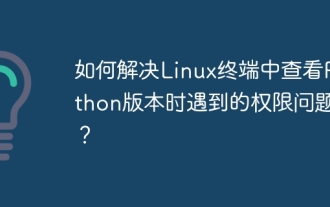
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
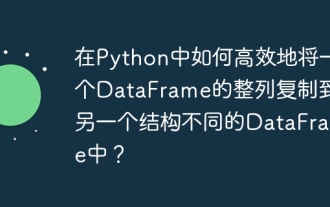
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
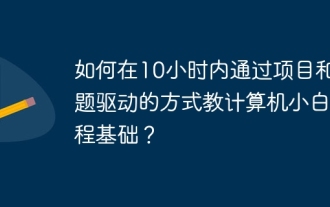
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
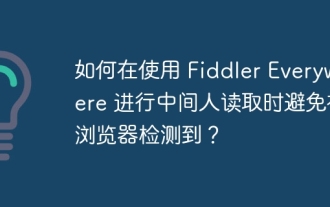
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
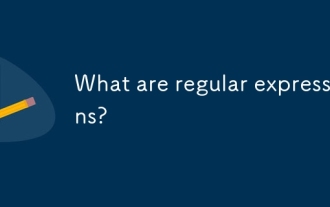
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
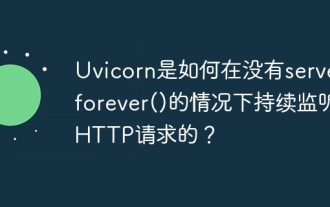
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
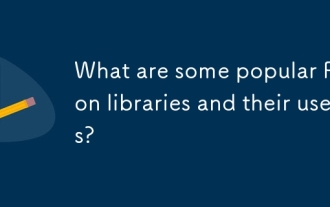
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
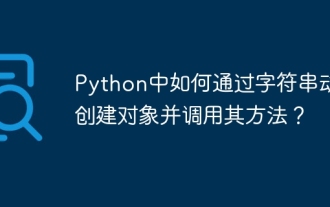
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
