How to Generate a Reversed IntStream in Java?
How to Reverse a Java Stream to Generate a Decreasing IntStream
Reversing a stream is a common operation when dealing with Java 8 streams. Here, we explore two approaches to reverse a stream of any type, and specifically address the challenge of generating a decreasing IntStream.
General Approach: Reversing a Stream of Any Type
To reverse a stream regardless of the element type, two techniques can be employed:
- Storing Elements in an Array: This method involves converting the stream into an array, then reading the elements backwards. However, it requires an unchecked cast due to the unknown runtime type of the stream elements.
- Using Collectors: This approach accumulates the stream elements into a reversed list, but involves frequent insertions at the front of ArrayList objects, resulting in copying overhead.
Specific Approach: Generating a Decreasing IntStream
The specific issue of generating a decreasing IntStream can be addressed with the following custom method:
<code class="java">static IntStream revRange(int from, int to) { return IntStream.range(from, to) .map(i -> to - i + from - 1); }</code>
This technique avoids boxing and sorting operations, resulting in more efficient code.
Additional Considerations
A previous version of this answer recommended using a reversed ArrayList. However, due to the inefficiency of inserting at the front of ArrayList, it has been updated to use an ArrayDeque instead, which supports efficient front insertions.
The output of this approach is a Deque instead of a List, but it can be easily iterated or streamed in the now-reversed order.
The above is the detailed content of How to Generate a Reversed IntStream in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










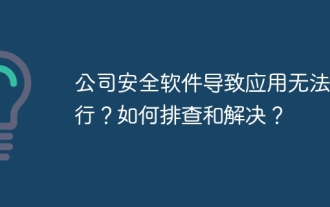
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
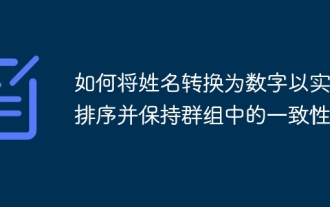
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
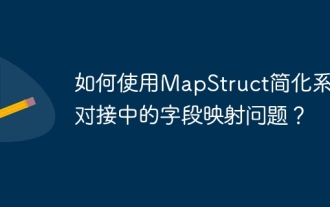
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
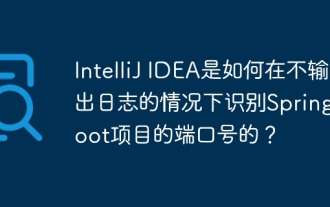
Start Spring using IntelliJIDEAUltimate version...
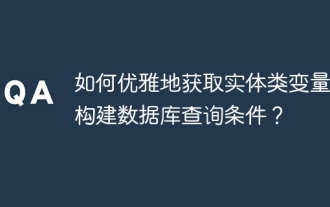
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
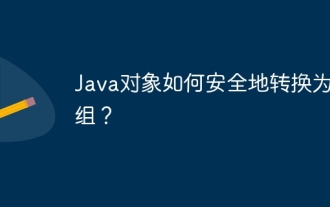
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
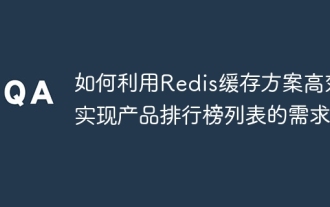
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
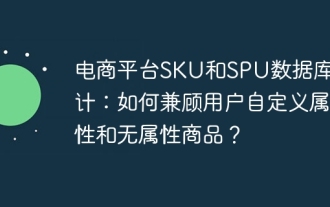
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
