


How to Properly Deallocate Memory Allocated with \'placement new\' in C ?
Proper Cleanup for Manually Allocated Memory: "placement new" and "delete"
In C , memory allocation and deallocation can be managed manually using the "placement new" and "delete" operators. This allows for greater flexibility in memory management, but also introduces potential pitfalls. Let's delve into the correct approach to deallocating memory allocated using "placement new."
Consider the code snippets below:
<code class="cpp">const char* charString = "Hello, World"; void *mem = ::operator new(sizeof(Buffer) + strlen(charString) + 1); Buffer* buf = new(mem) Buffer(strlen(charString)); delete (char*)buf;</code>
<code class="cpp">const char* charString = "Hello, World"; void *mem = ::operator new(sizeof(Buffer) + strlen(charString) + 1); Buffer* buf = new(mem) Buffer(strlen(charString)); delete buf;</code>
In both examples, memory is allocated using the low-level operator new function and cast to a void*. Then, a Buffer object is constructed in the allocated memory using the "placement new" syntax.
However, the crucial difference lies in the deallocation. The first snippet incorrectly attempts to delete the buf pointer as a char*, assuming the memory block was allocated using operator new. However, this will lead to memory corruption.
The second snippet demonstrates the correct approach:
<code class="cpp">buf->~Buffer(); ::operator delete(mem);</code>
When using "placement new," you must also manually call the destructor. Then, you should call the operator delete function, providing the same pointer as used with operator new. This ensures that both the object is destroyed properly and the allocated memory is freed back to the system.
Remember, only delete what you allocate. Directly calling operator new requires manually calling operator delete and the destructor for complete memory cleanup.
The above is the detailed content of How to Properly Deallocate Memory Allocated with \'placement new\' in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


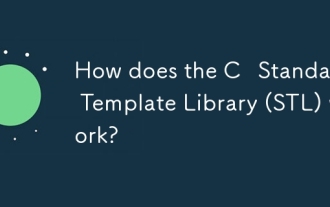
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
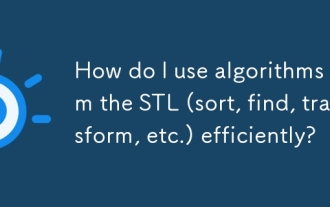
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
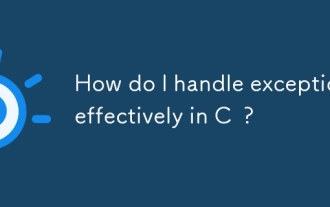
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
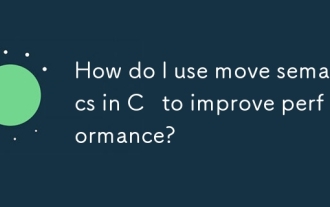
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
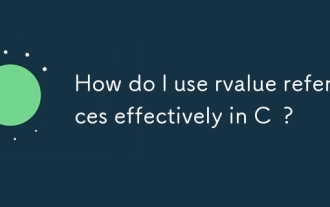
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
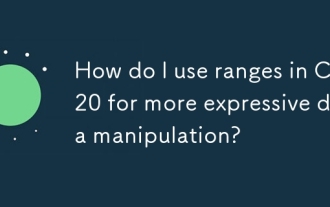
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
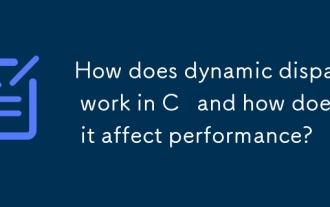
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
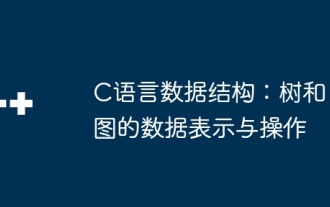
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
