


How Can You Efficiently Detect File Changes on an NTFS Volume Using FSCTL_ENUM_USN_DATA?
Efficiently Detecting File Changes on an NTFS Volume Using FSCTL_ENUM_USN_DATA
Background
Existing backup methods, which check each file's archive bit, can become slow and inefficient for large file systems. This approach requires scanning all files, including temporary files, and may result in lengthy backup processes.
Alternative Approach using File System USN
A more efficient method involves using the File System USN (Update Sequence Number) change journal. Filesystem USN provides a record for each change made to the file system, including file creation, deletion, and modification.
How FSCTL_ENUM_USN_DATA Works
To detect changes on an NTFS volume, we can utilize the FSCTL_ENUM_USN_DATA control code. This control code:
- Enumerates all files on a volume, including only currently existing ones.
-
Retrieves critical data for each file, including:
- File flags
- USN
- File names
- Parent file reference numbers
Implementing Change Detection
To detect changes:
- Obtain File System USN Data: Use FSCTL_QUERY_USN_JOURNAL to get the system's maximum USN (maxusn).
- Enumerate USN Records: Use a loop to iterate through USN records using FSCTL_ENUM_USN_DATA.
- Identify Relevant Records: Check flags and compare USNs to detect created, deleted, or modified files.
- Resolve Parent Paths: Match parent file reference numbers with file reference numbers of directories to obtain complete file paths.
Code Example in C
Here's a code snippet that demonstrates the approach:
<code class="c++">DWORDLONG nextid; DWORDLONG filecount = 0; DWORD starttick, endtick; // Allocate memory for USN records void * buffer = VirtualAlloc(NULL, BUFFER_SIZE, MEM_RESERVE | MEM_COMMIT, PAGE_READWRITE); // Open volume handle HANDLE drive = CreateFile(L"\\?\c:", GENERIC_READ, FILE_SHARE_DELETE | FILE_SHARE_READ | FILE_SHARE_WRITE, NULL, OPEN_ALWAYS, FILE_FLAG_NO_BUFFERING, NULL); // Get volume USN journal data USN_JOURNAL_DATA * journal = (USN_JOURNAL_DATA *)buffer; if (!DeviceIoControl(drive, FSCTL_QUERY_USN_JOURNAL, NULL, 0, buffer, BUFFER_SIZE, &bytecount, NULL)) { (...) } maxusn = journal->MaxUsn; MFT_ENUM_DATA mft_enum_data; mft_enum_data.StartFileReferenceNumber = 0; mft_enum_data.LowUsn = 0; mft_enum_data.HighUsn = maxusn; while (...) { if (!DeviceIoControl(drive, FSCTL_ENUM_USN_DATA, &mft_enum_data, sizeof(mft_enum_data), buffer, BUFFER_SIZE, &bytecount, NULL)) { (...) } nextid = *((DWORDLONG *)buffer); USN_RECORD * record = (USN_RECORD *)((USN *)buffer + 1); USN_RECORD * recordend = (USN_RECORD *)(((BYTE *)buffer) + bytecount); while (record < recordend) { filecount++; // Check flags and USNs to identify changes (...) record = (USN_RECORD *)(((BYTE *)record) + record->RecordLength); } mft_enum_data.StartFileReferenceNumber = nextid; }</code>
Performance Considerations
The approach using FSCTL_ENUM_USN_DATA offers:
- Fast enumeration process: Capable of processing over 6000 records per second.
- Efficient filtering: Only relevant file change records are analyzed, eliminating overhead from temporary files.
- Potential limitations: Performance may vary on very large volumes, but it is generally more efficient than checking archive bits.
Additional Notes
- Replace MFT_ENUM_DATA with MFT_ENUM_DATA_V0 on Windows versions later than Windows 7.
- File reference numbers are printed as 32-bit, which is a mistake. In production code, it's recommended to use 64-bit values.
The above is the detailed content of How Can You Efficiently Detect File Changes on an NTFS Volume Using FSCTL_ENUM_USN_DATA?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










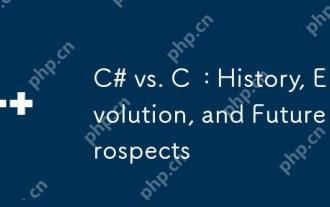
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
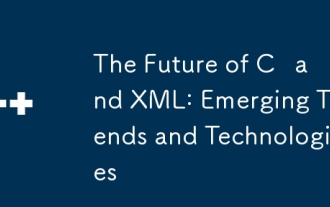
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
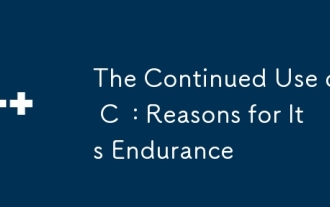
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
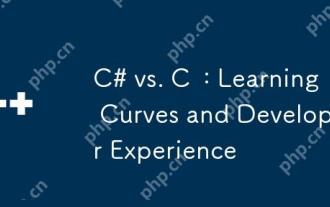
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
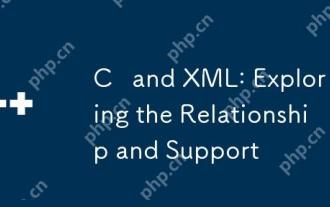
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
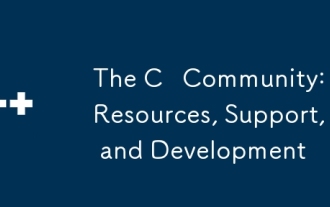
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
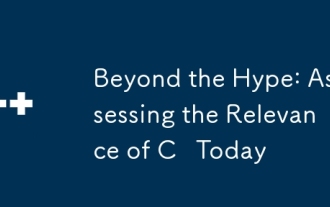
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
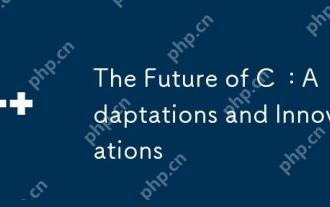
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
