Why Does `rand.Intn()` in Go Generate the Same Number Every Time?
Understanding Identical Random Number Generation in Go's rand.Intn()
Why does rand.Intn() consistently generate the same random number? This question often arises for beginners using Go due to the following code:
<code class="go">package main import ( "fmt" "math/rand" ) func main() { fmt.Println(rand.Intn(10)) }</code>
Despite the documentation claiming to provide a pseudo-random number in the range [0, n), the program prints the same number every time.
Seeding the Random Number Generation
The key lies in properly seeding the random number generator. The default behavior is to use a deterministic seed, resulting in the same sequence of numbers across program executions. To rectify this:
<code class="go">rand.Seed(time.Now().UnixNano())</code>
This function initializes the random number generator with a seed based on the current Unix timestamp. It ensures a different sequence of numbers for each run.
Understanding the Documentation
The documentation for rand.Intn() states: "Top-level functions ... produce a deterministic sequence of values each time a program is run." Therefore, without explicit seeding, the generator acts as if seeded by 1, leading to predictable results.
Conclusion
By seeding the random number generator before using rand.Intn(), you can overcome the issue of repeating random numbers, ensuring proper generation of non-deterministic sequences.
The above is the detailed content of Why Does `rand.Intn()` in Go Generate the Same Number Every Time?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










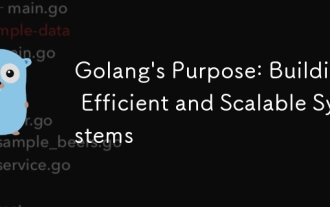
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
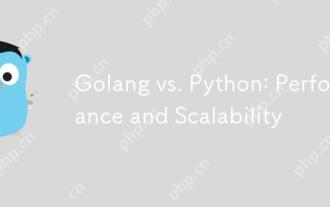
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
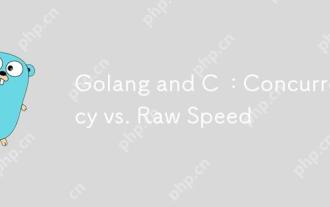
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
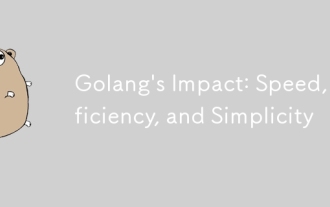
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
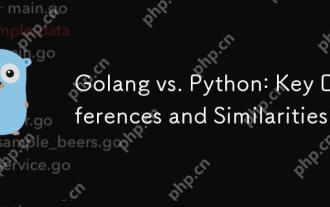
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
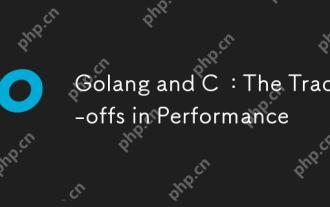
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
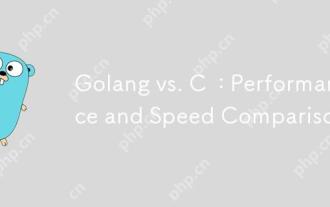
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
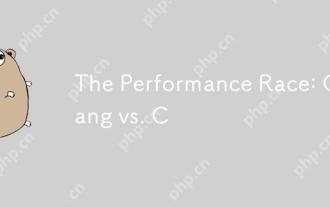
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
