


How Can You Avoid NameErrors When Calling Functions Defined Later in Python?
Forward-Declaring Functions to Prevent NameErrors
Encountering NameError exceptions when attempting to call functions defined later in the code can be frustrating. While Python's order of definition generally prohibits function usage before their declaration, this limitation can be circumvented using specific techniques.
For instance, to sort a list using a custom cmp_configs function that hasn't been defined yet, Python's immediate function feature can be employed:
<code class="python">print("\n".join([str(bla) for bla in sorted(mylist, cmp=cmp_configs)])) def cmp_configs(x, y): ... # Function implementation</code>
In this scenario, the sorted function call is wrapped within a separate function, resolving the immediate need for the cmp_configs definition. When the outer function is called, both sorted and cmp_configs will have been defined, ensuring proper execution.
Another common situation where forward-declaring functions is necessary is in recursion. Consider the following example:
<code class="python">def spam(): if end_condition(): return end_result() else: return eggs() def eggs(): if end_condition(): return end_result() else: return spam()</code>
When encountering this recursion pattern, one might assume that moving the eggs definition before spam would solve the issue. However, due to the circular dependency between the two functions, this approach still results in a NameError.
To address this specific situation, the custom function can be placed within the eggs function itself:
<code class="python">def eggs(): if end_condition(): return end_result() else: def spam(): if end_condition(): return end_result() else: return eggs() return spam() # Explicitly calling the inner 'spam' function</code>
Alternatively, the same result can be achieved using lambda expressions:
<code class="python">def eggs(): if end_condition(): return end_result() else: return lambda: spam() # Returns a callable object that implements spam</code>
In summary, while generally adhering to the principle of function definitions preceding their usage, there are scenarios where forward-declaring functions is unavoidable. By utilizing immediate functions or lambda expressions, programmers can circumvent these limitations and maintain the desired code structure without compromising functionality.
The above is the detailed content of How Can You Avoid NameErrors When Calling Functions Defined Later in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










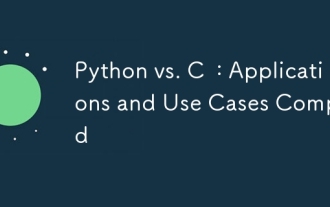
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
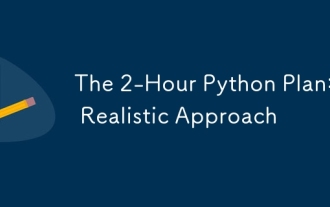
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
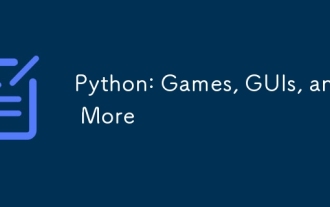
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
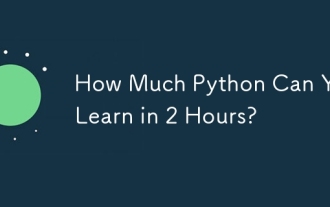
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
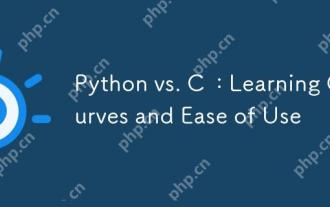
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
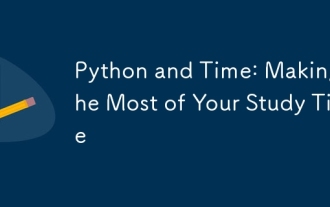
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
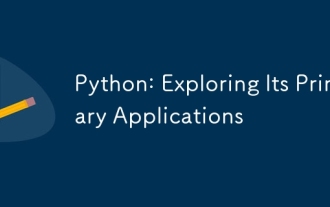
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
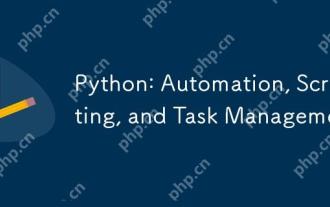
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
