


How can I programmatically create and manipulate INI files in PHP?
Creating and Manipulating INI Files in PHP
PHP offers limited built-in functionality for managing INI files. This can be a challenge when you need to create new files or modify existing ones programmatically.
However, a useful solution can be found in the PHP documentation comments. Here's a code snippet that addresses this issue:
<code class="php">function write_ini_file($assoc_arr, $path, $has_sections=FALSE) { // Generate content based on the provided data structure $content = ""; if ($has_sections) { // Handle sections and key-value pairs foreach ($assoc_arr as $key => $elem) { $content .= "[$key]\n"; foreach ($elem as $key2 => $elem2) { if (is_array($elem2)) { // Handle arrays within sections for ($i = 0; $i < count($elem2); $i++) { $content .= "$key2[] = \"$elem2[$i]\"\n"; } } elseif ($elem2 === "") { // Handle empty key-value pairs within sections $content .= "$key2 = \n"; } else { // Handle non-empty key-value pairs within sections $content .= "$key2 = \"$elem2\"\n"; } } } } else { // Handle flat INI file structure foreach ($assoc_arr as $key => $elem) { if (is_array($elem)) { // Handle arrays in flat structure for ($i = 0; $i < count($elem); $i++) { $content .= "$key[] = \"$elem[$i]\"\n"; } } elseif ($elem === "") { // Handle empty key-value pairs in flat structure $content .= "$key = \n"; } else { // Handle non-empty key-value pairs in flat structure $content .= "$key = \"$elem\"\n"; } } } // Write the generated content to the INI file if (!$handle = fopen($path, 'w')) { return false; // Unable to open the file } $success = fwrite($handle, $content); fclose($handle); return $success; // Return success status }
Usage:
<code class="php">$sampleData = array( 'first' => array( 'first-1' => 1, 'first-2' => 2, 'first-3' => 3, 'first-4' => 4, 'first-5' => 5, ), 'second' => array( 'second-1' => 1, 'second-2' => 2, 'second-3' => 3, 'second-4' => 4, 'second-5' => 5, ), ); write_ini_file($sampleData, './data.ini', true); // Write data to 'data.ini' with sections</code>
This solution allows you to create and manipulate INI files with ease in your PHP applications.
The above is the detailed content of How can I programmatically create and manipulate INI files in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










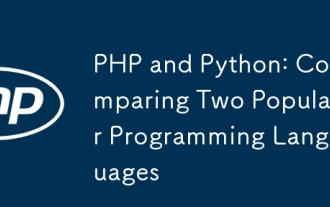
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
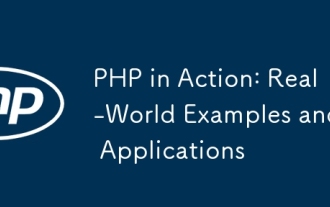
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
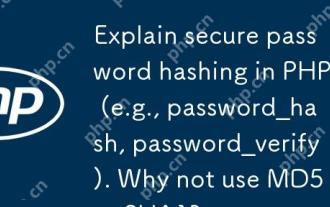
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
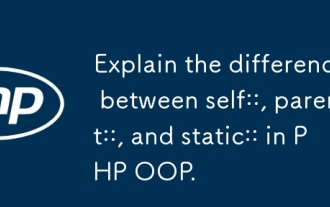
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
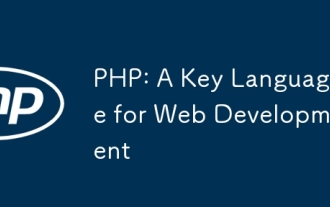
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
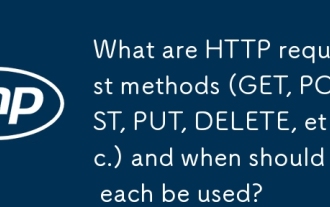
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
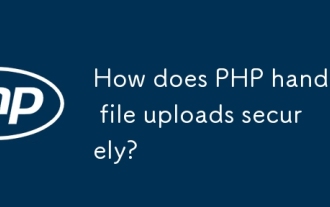
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
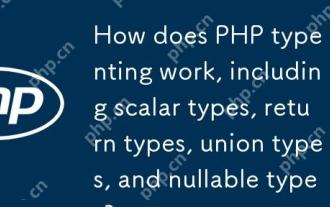
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
