


How to Correctly Pass Parameters to .Call Function in the reflect Package?
Understanding .Call in the Reflect Package
When utilizing the .Call function in the reflect package, it's essential to comprehend how to correctly manipulate the "in" variable to pass appropriate function parameters.
Consider the following scenario:
<code class="go">params := "some map[string][]string" in := make([]reflect.Value, 0) return_values := reflect.ValueOf(&controller_ref).MethodByName(action_name).Call(in)</code>
The function being invoked is defined as:
<code class="go">func (c *Controller) Root(params map[string][]string) map[string] string{}</code>
To resolve the error "reflect: Call with too few input arguments," it's crucial to understand the syntax of .Call. As stated in its documentation, each element in the in slice corresponds to a function argument. Therefore, if your function requires a single parameter, in should contain one reflect.Value of the appropriate type.
In the provided example, the correct approach is:
<code class="go">m := map[string][]string{"foo": []string{"bar"}} in := []reflect.Value{reflect.ValueOf(m)} myMethod.Call(in)</code>
This line creates a map, converts it to a reflect.Value, and passes it as the sole argument to the function call.
The above is the detailed content of How to Correctly Pass Parameters to .Call Function in the reflect Package?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


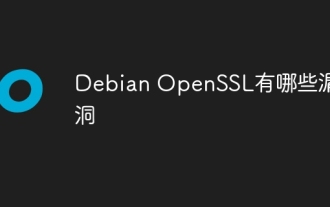
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
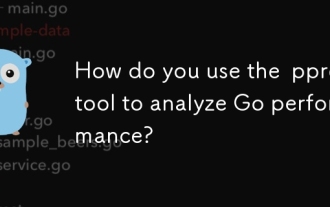
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
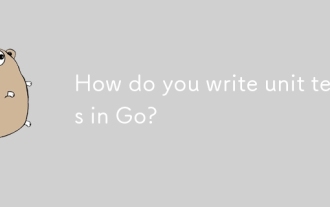
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
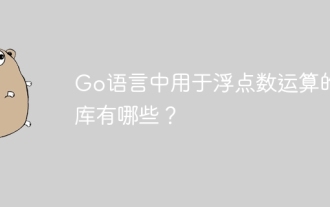
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
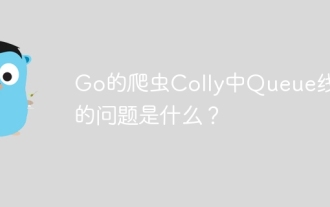
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
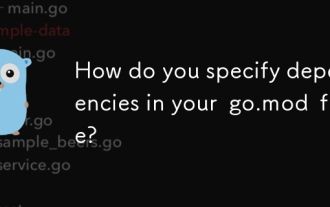
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
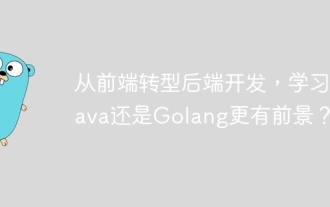
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
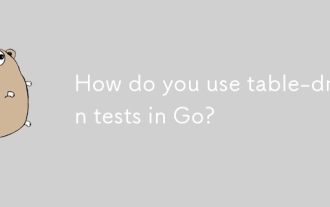
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
