


How to Safely Delete Items from a Dictionary During Iteration in Python?
Deleting Items from a Dictionary While Iterating in Python
When working with dictionaries in Python, there may be instances where you need to remove items that fulfill specific criteria during iteration. While intuitively, it might seem that iterating directly over the dictionary and deleting items as encountered would suffice, this approach is problematic.
Challenges with Direct Deletion during Iteration
In Python 3, directly deleting items from a dictionary while iterating over it raises a RuntimeError. This is because modifying the dictionary size during iteration causes the iterator to malfunction.
In Python 2, this direct deletion approach results in an error if the item to be deleted is the next item in the iteration sequence.
Solutions
To safely delete items from a dictionary while iterating, here are recommended solutions:
Python 3 :
Convert the dictionary keys to a list using list(mydict.keys()) before iteration. This allows you to iterate over the list while making changes to the dictionary:
<code class="python">for k in list(mydict.keys()): if mydict[k] == 3: del mydict[k]</code>
Python 2:
Utilize the keys() or items() methods to create a list of keys or tuples of key-value pairs, respectively. You can then safely iterate over these lists to remove items without disrupting the iterator:
<code class="python">for k in mydict.keys(): if k == 'two': del mydict[k]</code>
<code class="python">for k, v in mydict.items(): if v == 3: del mydict[k]</code>
The above is the detailed content of How to Safely Delete Items from a Dictionary During Iteration in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
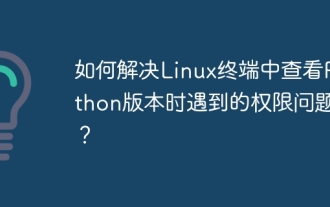
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
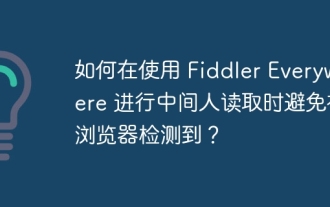
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
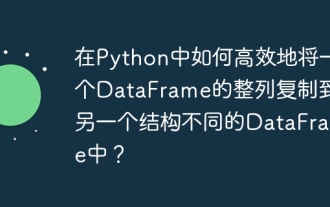
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
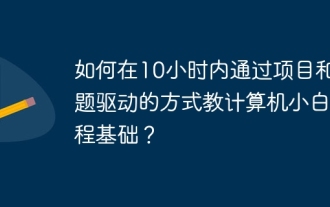
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
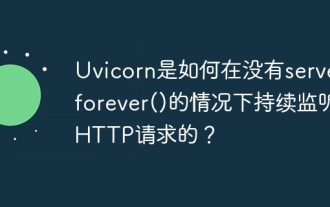
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
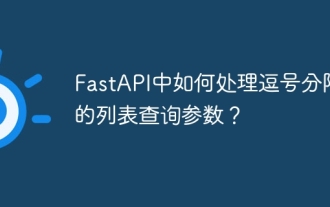
Fastapi ...
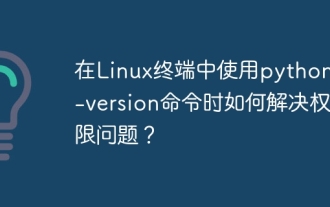
Using python in Linux terminal...
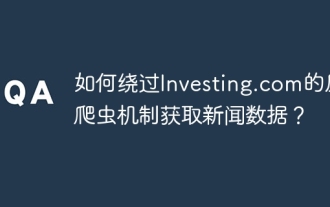
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
