


How to Fix \'no kind \'Deployment\' is registered for version \'apps/v1beta1\'\' Error When Deserializing Kubernetes YAML?
How to Deserialize Kubernetes YAML File
Issue
You've encountered an error when attempting to deserialize a Kubernetes YAML file into a Go struct using the api.Codecs.UniversalDecoder().Decode function. The error reads, "no kind "Deployment" is registered for version "apps/v1beta1."
Understanding the Problem
When deserializing a Kubernetes YAML file, you must ensure that the schema of the object is registered. In this case, the Deployment object is registered under the apps/v1beta1 version of the API.
Solution
To resolve the issue, you need to import the package that registers the schema for the apps/v1beta1 version. This can be achieved by adding the following line to your code:
<code class="go">_ "k8s.io/client-go/pkg/apis/extensions/install"</code>
This import ensures that the schema for the Deployment object is registered and available for use during the deserialization process.
Working Example
Here's a modified working Go program that incorporates the necessary import:
<code class="go">package main import ( "fmt" "k8s.io/client-go/pkg/api" "k8s.io/client-go/pkg/api/install" "k8s.io/client-go/pkg/apis/extensions/install" ) var service = ` apiVersion: apps/v1beta1 kind: Deployment metadata: name: my-nginx spec: replicas: 2 template: metadata: labels: run: my-nginx spec: containers: - name: my-nginx image: nginx ports: - containerPort: 80 ` func main() { decode := api.Codecs.UniversalDecoder().Decode obj, _, err := decode([]byte(service), nil, nil) if err != nil { fmt.Printf("%#v", err) } fmt.Printf("%#v\n", obj) }</code>
When you run this program, the Deployment object should be successfully deserialized without encountering the aforementioned error.
The above is the detailed content of How to Fix \'no kind \'Deployment\' is registered for version \'apps/v1beta1\'\' Error When Deserializing Kubernetes YAML?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










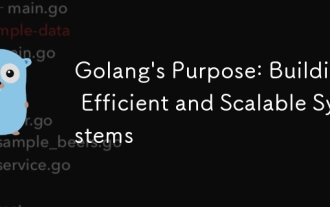
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
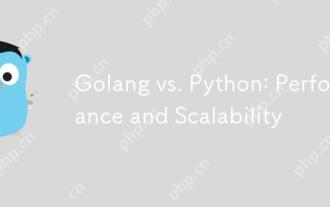
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
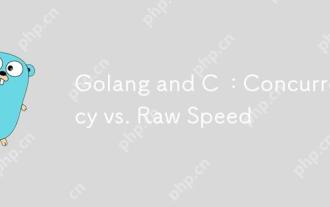
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
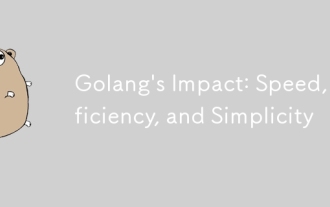
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
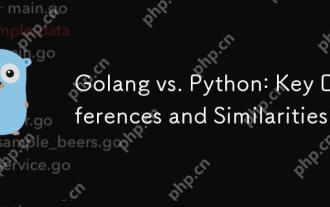
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
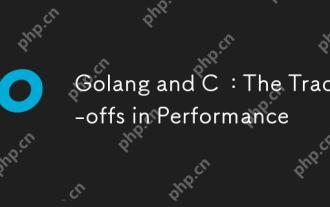
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
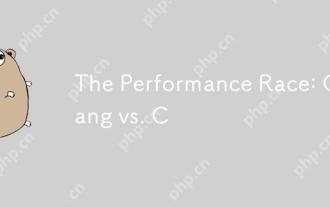
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
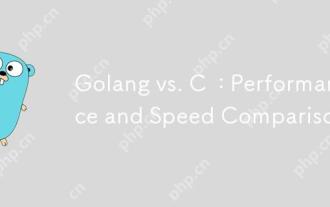
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
