


How to retrieve the class name from a static method call in extended PHP classes?
Retrieving Class Name from Static Call in Extended PHP Class
In the world of PHP, it's often necessary to determine the classname from a static function call, especially when working with extended classes. Consider the following scenario:
<code class="php">class Action { function n() {/* some implementation */} } class MyAction extends Action {/* further implementation */}</code>
In this situation, calling MyAction::n(); should return "MyAction" as the classname. However, the __CLASS__ variable only returns the parent class name ("Action").
Late Static Bindings (PHP 5.3 ):
PHP 5.3 introduced late static bindings, which enable resolving the target class at runtime. This feature makes it possible to determine the called class using the get_called_class() function:
<code class="php">class Action { public static function n() { return get_called_class(); } } class MyAction extends Action { } echo MyAction::n(); // Output: MyAction</code>
Alternative Approach (Pre-PHP 5.3):
Prior to PHP 5.3, an alternative solution relies on using a non-static method and the get_class() function:
<code class="php">class Action { public function n(){ return get_class($this); } } class MyAction extends Action { } $foo = new MyAction; echo $foo->n(); // Output: MyAction</code>
Remember, this approach only works for non-static methods, as the get_class() function takes an instance of the class as an argument.
The above is the detailed content of How to retrieve the class name from a static method call in extended PHP classes?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


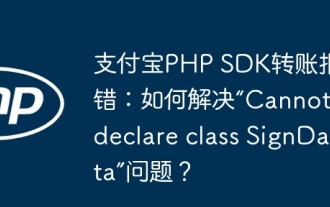
Alipay PHP...
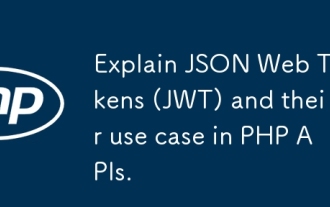
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
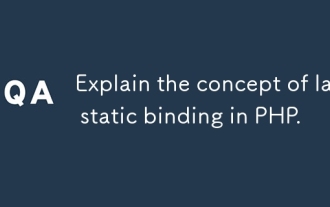
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
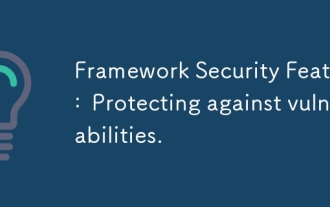
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
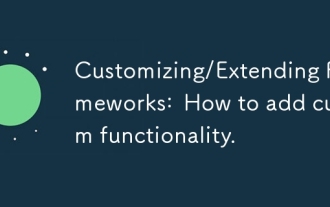
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
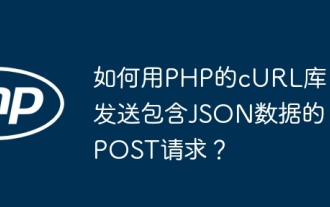
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
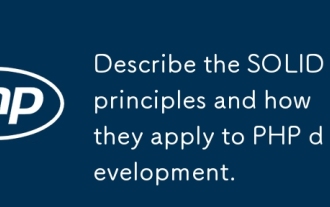
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
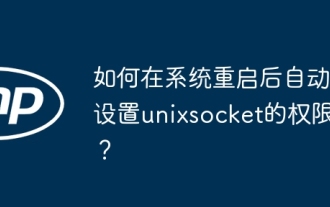
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
