How to Track Upload Progress for POST Requests in Go?
Achieving Upload Progress Tracking for POST Requests in Go
In the quest to create a ShareX clone for Linux that seamlessly uploads files via HTTP POST requests, a critical question arises: how to effectively track upload progress, especially for larger files that may take minutes to transfer?
While manually establishing a TCP connection and sending HTTP requests in chunks is a viable solution, it carries limitations when dealing with HTTPS sites and may not be the most efficient approach. Fortunately, Go offers an alternative that effortlessly integrates progress tracking into your request process.
Customizing io.Reader for Progress Monitoring
The key insight lies in creating a custom io.Reader that wraps the actual reader. By overriding the Read() method, you can capture the progress at each Read invocation.
Consider the following code snippet:
1 2 3 4 5 6 7 8 9 10 |
|
In this structure, Reporter is a callback function that receives the number of bytes read as an argument. The ProgressReader then intercepts the Read operation and reports the progress to the callback.
Implementation in Practice
To put this concept into practice, you can employ the following steps:
-
Create an instance of ProgressReader, wrapping your file reader:
1
2
file, _ := os.Open(
"/tmp/blah.go"
)
pr := &ProgressReader{file, func(r int64) { ... }}
Copy after login -
Define the Reporter function to handle progress updates:
1
2
3
4
5
6
7
8
pr.Reporter = func(r int64) {
total += r
if
r > 0 {
fmt.Println(
"progress"
, r)
}
else
{
fmt.Println(
"done"
, r)
}
}
Copy after login -
Perform the file copy using your custom reader:
1
io.
Copy
(ioutil.Discard, pr)
Copy after login
By utilizing this approach, you can effortlessly monitor upload progress for POST requests in Go, providing users with real-time insights into the file transfer process.
The above is the detailed content of How to Track Upload Progress for POST Requests in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










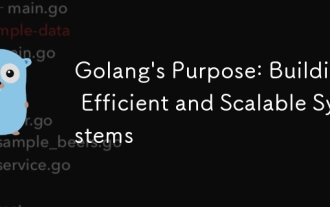
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
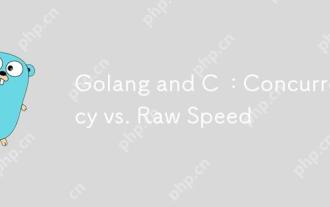
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
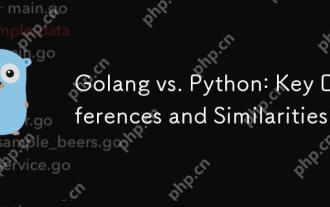
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
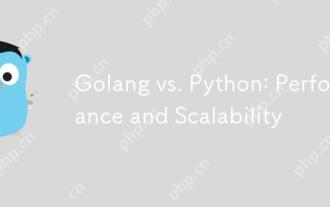
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
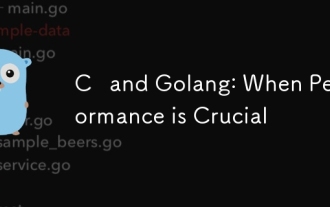
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
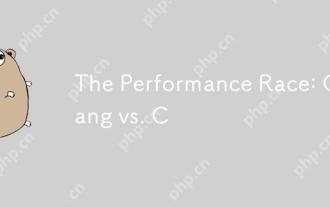
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
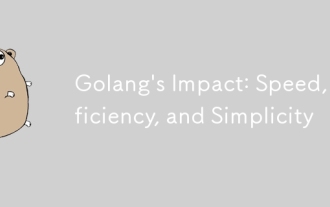
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
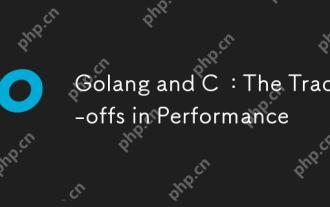
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
