When to Use `Optional.orElseGet()` Over `Optional.orElse()`?
Difference between Optional.orElse() and Optional.orElseGet()
The Optional class in Java provides convenient methods for handling and returning values that may or may not be present. Two important methods in this class are orElse() and orElseGet(). The primary difference between these methods lies in the timing of when the fallback value or computation is invoked.
orElse()
The orElse() method takes a default value as an argument and returns that value if the Optional instance is empty. It does not wait for isPresent() to be evaluated, but immediately evaluates and returns the provided fallback value, even if the Optional instance is not empty.
orElseGet()
In contrast, the orElseGet() method takes a Supplier interface as an argument, allowing you to define a computation that only runs when the Optional instance is empty. This approach is more efficient when the fallback value is expensive to compute and should only be performed when necessary.
When to Use orElseGet() Over orElse()
Consider using orElseGet() instead of orElse() in the following scenarios:
- Performance Optimization: orElseGet() ensures that the fallback computation is only performed when needed, improving performance if the fallback computation is resource-intensive.
- Exception Handling: If the fallback computation can throw an exception, orElseGet() allows you to wrap it in a more controlled manner, avoiding any unexpected exceptions from being thrown.
- Code Readability: Using orElseGet() with a descriptive Supplier can improve code readability by indicating when the fallback computation is being executed.
Example Usage
Consider the following example:
<code class="java">Optional<String> optional = Optional.ofNullable("Hello"); // Always computes the fallback value. String result1 = optional.orElse("World"); // Computes the fallback value only if the optional is empty. String result2 = optional.orElseGet(() -> { // Expensive computation here return "World"; });</code>
In this example, result1 will always contain "World" because the fallback value is evaluated regardless of the option being present. On the other hand, result2 will only be assigned "World" if the optional is empty, allowing for better performance when the computation is expensive.
The above is the detailed content of When to Use `Optional.orElseGet()` Over `Optional.orElse()`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


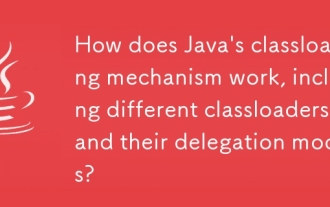
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
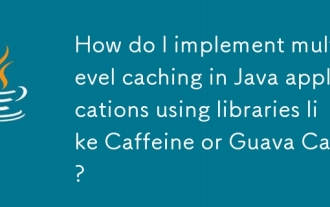
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
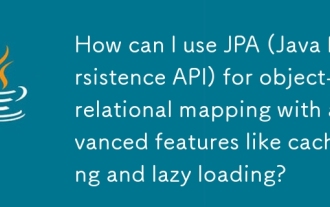
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
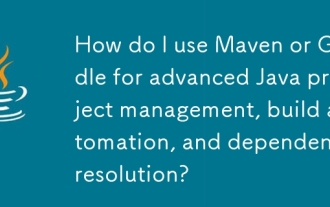
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
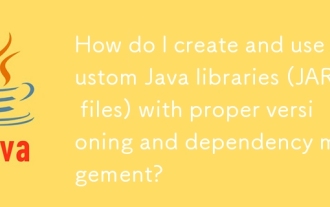
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
