


How to Calculate the Difference in Scores for Multiple Fields in a Pandas DataFrame?
Pandas groupby on Multiple Fields with Difference Calculation
In programming, manipulating data is crucial, and Pandas is a powerful library for performing these tasks efficiently. One common question is how to group data by multiple fields and calculate differences. Let's explore how to achieve this.
Problem:
Consider a DataFrame with the following structure:
date site country score 0 2018-01-01 google us 100 1 2018-01-01 google ch 50 2 2018-01-02 google us 70 3 2018-01-03 google us 60 ...
The goal is to find the 1/3/5-day difference in scores for each 'site/country' combination.
Solution:
To solve this problem, we can utilize Pandas' groupby and diff functions:
- Sort the DataFrame:
df = df.sort_values(by=['site', 'country', 'date'])
Sorting ensures that our data is organized for proper grouping and difference calculations.
- Groupby and Calculate Difference:
df['diff'] = df.groupby(['site', 'country'])['score'].diff().fillna(0)
This line groups the DataFrame by 'site' and 'country' columns using groupby. Then, it calculates the difference between each consecutive score within each group using diff. The result is stored in a new column called 'diff.' Any missing values are replaced with 0 using fillna(0).
Output:
The resulting DataFrame will contain the original columns along with the 'diff' column:
date site country score diff 0 2018-01-01 fb es 100 0.0 1 2018-01-02 fb gb 100 0.0 ...
Additional Notes:
- If you require arbitrary sorting (e.g., prioritizing 'google' over 'fb'), you can specify the order in a list and set the column as categorical before sorting.
- The fillna(0) function replaces missing values with 0, but you can change this to any desired value.
- This method can be used to calculate differences over any time interval (e.g., 1-day, 3-month, etc.).
The above is the detailed content of How to Calculate the Difference in Scores for Multiple Fields in a Pandas DataFrame?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










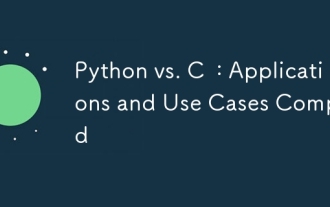
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
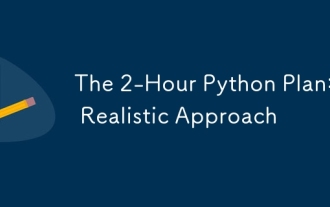
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
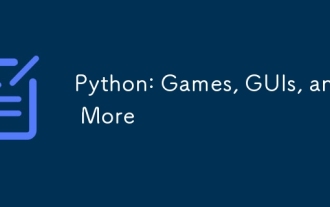
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
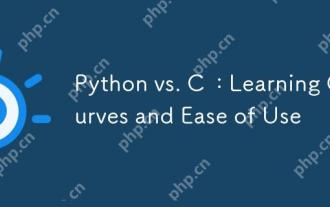
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
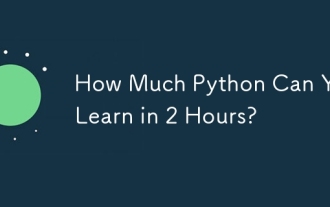
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
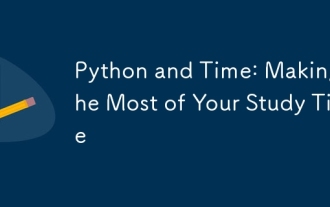
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
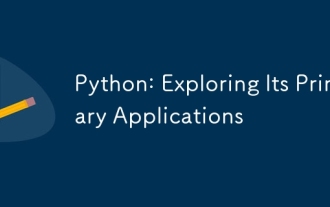
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
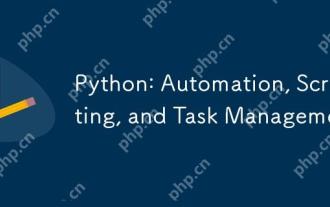
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
