


How to Establish Cross-Module Variable Accessibility in Python: Sharing Variables Without Sharing Instances?
Establishing Cross-Module Variable Accessibility
In Python, a convenient cross-module variable is __debug__. However, creating a custom variable with similar functionality may seem challenging. This article delves into this topic, exploring a method to define a shared variable across modules while preserving its immutability.
Solution: Leveraging Global Module-Level Variables
To establish a cross-module variable without sharing a common variable instance, consider employing global module-level variables. These variables are defined and assigned within a module and become accessible to other modules that import it. By assigning the variable before importing dependent modules, a consistent value can be established throughout the project.
An example is provided below:
a.py:
1 |
|
b.py:
1 2 3 4 |
|
c.py:
1 2 |
|
Test:
1 2 3 4 |
|
In this example, variable var is defined in module a.py and becomes accessible to module b.py upon its import. Even though module c.py changes the value of var, module b.py retains its original value.
Real-World Application
Django provides a practical use case for shared module-level variables. Its global_settings.py module serves as an example where settings are exposed as module attributes rather than a single shared object.
By employing this technique, cross-module variable sharing can be achieved while maintaining variable integrity and simplifying module code organization.
The above is the detailed content of How to Establish Cross-Module Variable Accessibility in Python: Sharing Variables Without Sharing Instances?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
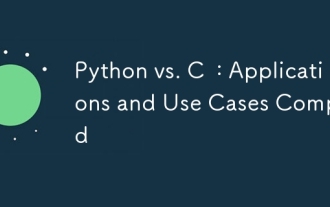
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
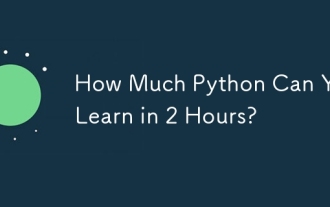
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
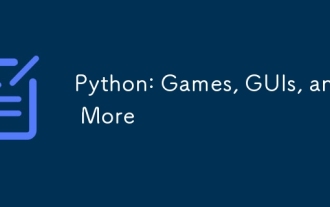
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
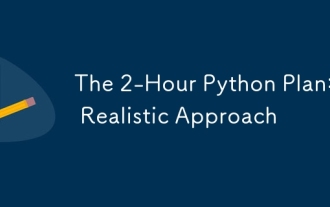
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
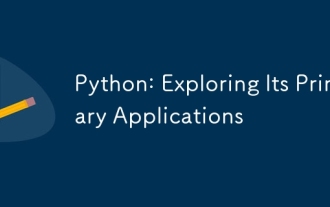
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
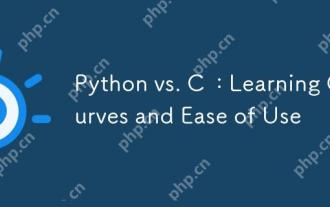
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
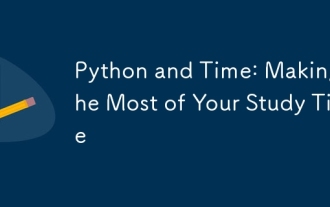
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
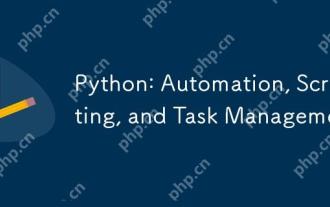
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
