Do HTTP Requests Automatically Retry on Server Outages?
HTTP Request Retrying Mechanism
Q: I'm attempting to push data to an Apache server using Go. If the Apache server experiences a temporary shutdown, will my HTTP request automatically retry?
A: No, HTTP requests do not inherently retry upon server outages.
Custom Retry Logic
To implement automatic retries, you need to create your own retry mechanism. A basic implementation is available at the following link:
[Go Retry Function Example](https://play.golang.org/p/_o5AgePDEXq)
<code class="go">package main import ( "fmt" "io/ioutil" "log" "net/http" ) func main() { var ( err error response *http.Response retries int = 3 ) for retries > 0 { response, err = http.Get("https://non-existent") if err != nil { log.Println(err) retries -= 1 } else { break } } if response != nil { defer response.Body.Close() data, err := ioutil.ReadAll(response.Body) if err != nil { log.Fatal(err) } fmt.Printf("data = %s\n", data) } }</code>
In this example, a custom retry mechanism is implemented using a loop that attempts an HTTP request multiple times before failing. You can adjust the number of retries and the logic for determining whether to retry based on the specific needs of your application.
The above is the detailed content of Do HTTP Requests Automatically Retry on Server Outages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
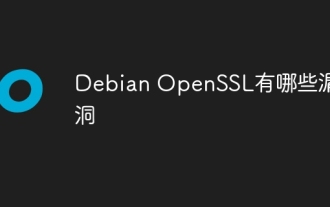
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
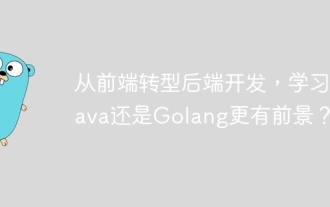
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
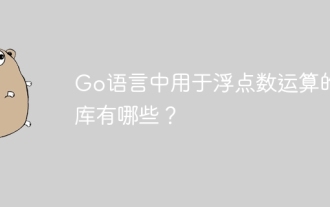
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
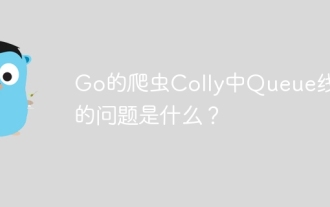
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
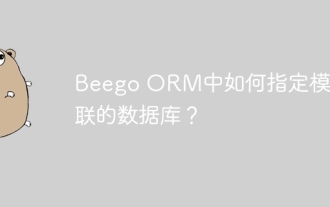
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
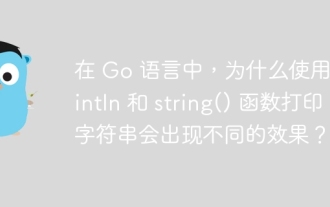
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
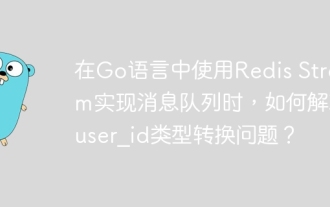
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
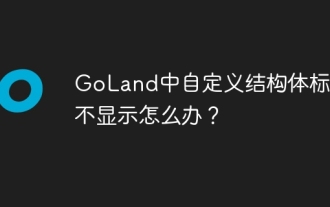
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
