How to Extract the Subject DN from an X509 Certificate in Go?
How to Extract Subject DN from X509 Certificate in Go
Retrieving the complete subject distinguished name (DN) from an X509 certificate in Go as a string can be challenging. Despite lacking a dedicated ".String()" method for the pkix.Name type, there is a multifaceted solution.
Solution:
The following function leverages a predefined map to translate OIDs into meaningful field names (e.g., "CN" for Common Name):
<code class="go">import ( "fmt" "strings" "crypto/x509" "crypto/x509/pkix" ) var oid = map[string]string{ "2.5.4.3": "CN", "2.5.4.6": "C", "2.5.4.7": "L", "2.5.4.8": "ST", "2.5.4.10": "O", "2.5.4.11": "OU", "1.2.840.113549.1.9.1": "emailAddress", } func getDNFromCert(namespace pkix.Name, sep string) (string, error) { subject := []string{} for _, s := range namespace.ToRDNSequence() { for _, i := range s { if v, ok := i.Value.(string); ok { if name, ok := oid[i.Type.String()]; ok { subject = append(subject, fmt.Sprintf("%s=%s", name, v)) } else { subject = append(subject, fmt.Sprintf("%s=%s", i.Type.String(), v)) } } else { subject = append(subject, fmt.Sprintf("%s=%v", i.Type.String(), v)) } } } return sep + strings.Join(subject, sep), nil }</code>
Usage:
To extract the subject DN, invoke the function as follows:
<code class="go">subj, err := getDNFromCert(x509Cert.Subject, "/") if err != nil { // Error handling } fmt.Println(subj)</code>
Example Output:
/C=US/O=some organization/OU=unit/CN=common name
The above is the detailed content of How to Extract the Subject DN from an X509 Certificate in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










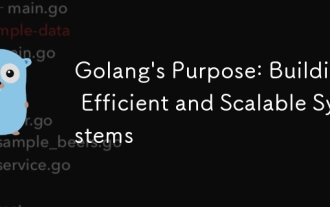
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
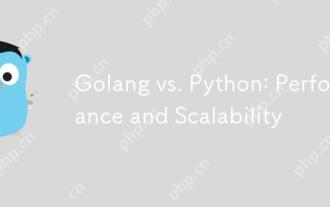
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
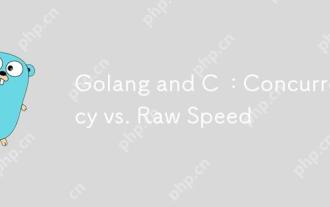
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
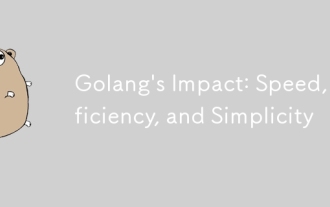
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
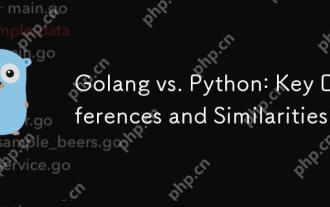
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
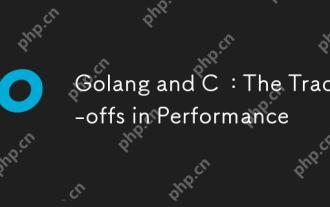
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
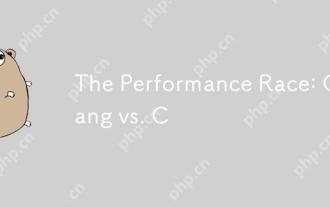
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
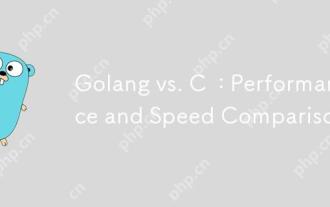
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
