How to Count Word Frequencies in Java 8 Using Streams?
Word Frequency Count in Java 8 Using Streams
Counting the frequency of words within a list is a common task in text processing. In Java 8, this can be achieved efficiently using the Collectors.groupingBy() and Collectors.counting() methods.
To find the word frequency in a list of strings, you can use the following steps:
- Call stream() on the list to create a stream.
- Group the elements in the stream by their values using Collectors.groupingBy(Function.identity()).
- Count the occurrences of each word using Collectors.counting().
For example, given the following list of words:
List<String> wordsList = Lists.newArrayList("hello", "bye", "ciao", "bye", "ciao");
You can calculate the word frequencies as follows:
Map<String,Long> wordFrequencies = wordsList.stream() .collect(Collectors.groupingBy(Function.identity(), Collectors.counting()));
This will produce a map with the word frequencies, where the keys are the words and the values are the number of occurrences. The result for the given list would be:
{ciao=2, hello=1, bye=2}
To sort the map by values, you can use LinkedHashMap and the entrySet() method to collect the map entries into a stream. Then, use sorted() to sort the entries by value in descending order and Collectors.toMap() to collect them into a new LinkedHashMap:
LinkedHashMap<String, Long> countByWordSorted = wordFrequencies.entrySet() .stream() .sorted(Map.Entry.comparingByValue(Comparator.reverseOrder())) .collect(Collectors.toMap( Map.Entry::getKey, Map.Entry::getValue, (v1, v2) -> { throw new IllegalStateException(); }, LinkedHashMap::new ));
This will produce a sorted map where the words are ordered by their frequency.
The above is the detailed content of How to Count Word Frequencies in Java 8 Using Streams?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




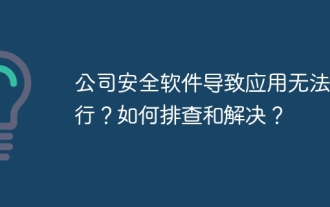
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
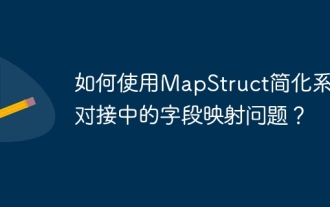
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
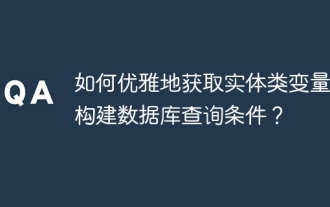
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
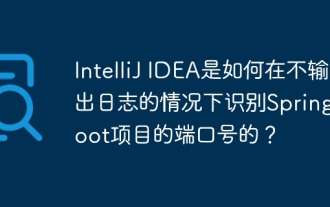
Start Spring using IntelliJIDEAUltimate version...
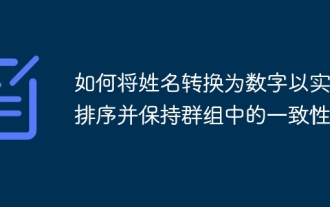
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
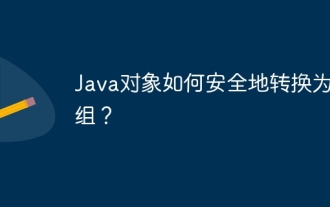
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
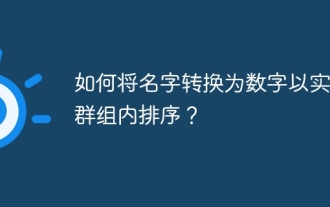
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
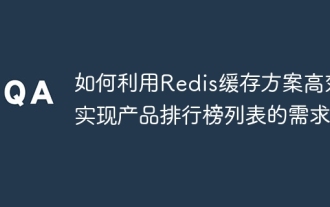
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
