How to Execute External JAR Files and Display Runtime Output in a Java GUI?
Executing External JAR Files in a Java GUI
Creating a Java GUI application that allows users to execute other JAR files with button clicks and display runtime process details can be accomplished through the following steps:
Run External JARs:
To execute external JAR files, create a separate Java process using Runtime.getRuntime().exec(). This function takes a command as a string and launches a new process on the operating system. For example, to execute A.jar, use the following code:
<code class="java">Process proc = Runtime.getRuntime().exec("java -jar A.jar");</code>
Retrieve Process Output:
After launching the JAR, you can retrieve its runtime output by accessing the process's input and error streams:
<code class="java">InputStream in = proc.getInputStream(); InputStream err = proc.getErrorStream();</code>
Display Output in GUI:
To display the output in your GUI, buffer the input and error streams using a BufferedReader and read the lines one by one. You can then add these lines to a text area or other display component in your GUI.
Example Code:
The following example code creates a simple GUI with two buttons that execute A.jar and B.jar respectively and displays the output in a text area:
<code class="java">import javax.swing.*; import java.awt.event.*; import java.io.*; public class JarExecutor extends JFrame { private JTextArea outputArea; private JButton buttonA, buttonB; public JarExecutor() { // ... // Setup GUI components // Create buttons buttonA = new JButton("A"); buttonA.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { executeJar("A.jar"); } }); buttonB = new JButton("B"); buttonB.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { executeJar("B.jar"); } }); // ... // Add buttons to GUI // Create text area for output outputArea = new JTextArea(); outputArea.setEditable(false); outputArea.setLineWrap(true); // ... // Add text area to GUI } private void executeJar(String jarPath) { try { Process proc = Runtime.getRuntime().exec("java -jar " + jarPath); BufferedReader in = new BufferedReader(new InputStreamReader(proc.getInputStream())); BufferedReader err = new BufferedReader(new InputStreamReader(proc.getErrorStream())); String line; while ((line = in.readLine()) != null) { outputArea.append(line + "\n"); } while ((line = err.readLine()) != null) { outputArea.append(line + "\n"); } } catch (IOException e) { outputArea.append("Error executing JAR: " + e.getMessage() + "\n"); } } // ... // Main method }</code>
The above is the detailed content of How to Execute External JAR Files and Display Runtime Output in a Java GUI?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










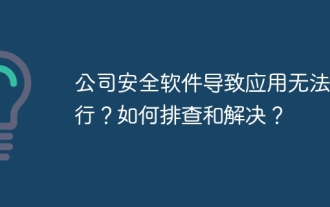
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
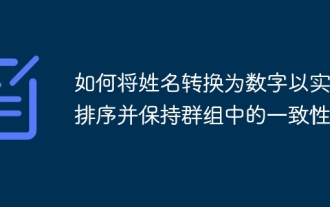
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
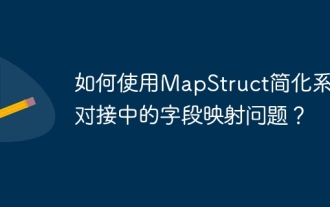
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
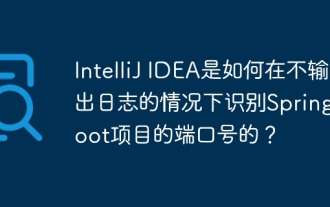
Start Spring using IntelliJIDEAUltimate version...
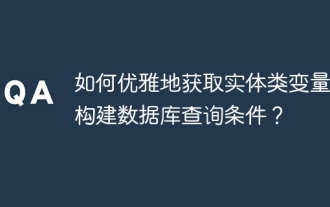
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
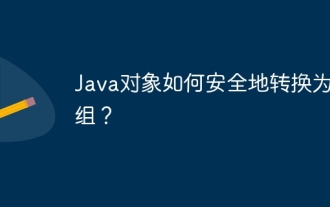
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
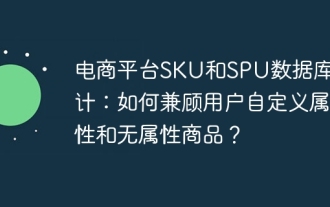
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
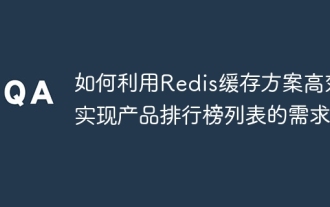
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
