


How to Remove and Add Items in an Android RecyclerView with a Cross Button for Deletion?
Android RecyclerView Item Removal and Addition
Android RecyclerView provides an efficient way to display large datasets and handle item insertion and removal. This article demonstrates how to handle item addition and removal from a RecyclerView, focusing on a scenario with a TextView and a cross button ImageView.
Problem:
A RecyclerView with a list of items is presented, and each item has a name and a cross button. The cross button should remove the corresponding item when pressed.
Solution:
- Implement a Custom Adapter (MyAdapter):
Extend the RecyclerView.Adapter class and define a ViewHolder class that holds references to the TextView and ImageView.
<code class="java">public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> { private ArrayList<String> mDataset; private static Context sContext; public MyAdapter(Context context, ArrayList<String> myDataset) { mDataset = myDataset; sContext = context; } @Override public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { // Create a view and ViewHolder ViewHolder holder = new ViewHolder(v); holder.mNameTextView.setOnClickListener(this); holder.mNameTextView.setOnLongClickListener(this); return holder; } @Override public void onBindViewHolder(ViewHolder holder, int position) { // Bind data to the ViewHolder holder.mNameTextView.setText(mDataset.get(position)); } @Override public int getItemCount() { return mDataset.size(); } @Override public void onClick(View view) { // Handle click events for the TextView Toast.makeText(sContext, holder.mNameTextView.getText(), Toast.LENGTH_SHORT).show(); } @Override public boolean onLongClick(View view) { // Handle long-click events for the TextView mDataset.remove(holder.getPosition()); notifyDataSetChanged(); return false; } public static class ViewHolder extends RecyclerView.ViewHolder { public TextView mNameTextView; public ImageView mCrossButtonImageView; public ViewHolder(View v) { super(v); mNameTextView = (TextView) v.findViewById(R.id.nameTextView); mCrossButtonImageView = (ImageView) v.findViewById(R.id.crossButton); } } }</code>
- Implement Click Event Handling for the Cross Button:
Override the onClick method in the ViewHolder to handle click events for the cross button ImageView.
<code class="java">@Override public void onClick(View v) { // Check if the view clicked is the cross button if (v.equals(holder.mCrossButtonImageView)) { // Remove the item from the dataset mDataset.remove(holder.getPosition()); // Notify the adapter of the item removal notifyItemRemoved(holder.getPosition()); } else { // Handle other click events if needed } }</code>
- Set the Visibility of the Cross Button ImageView:
To make the cross button ImageView initially hidden and display it when needed, use the setVisibility method in your onBindViewHolder.
<code class="java">@Override public void onBindViewHolder(ViewHolder holder, int position) { // Bind data to the ViewHolder holder.mNameTextView.setText(mDataset.get(position)); // Check if the cross button should be visible if (itemClicked) { holder.mCrossButtonImageView.setVisibility(View.VISIBLE); } else { holder.mCrossButtonImageView.setVisibility(View.GONE); } }</code>
- Additional Implementation Details:
- You can use a boolean flag to control the visibility of the cross button ImageView.
- Handle other desired click events (such as TextView clicks) by overriding the onClick method in your ViewHolder.
- Consider implementing "Undo" functionality to restore removed items if desired.
Conclusion:
This approach allows you to dynamically add and remove items from a RecyclerView and configure the visibility of a cross button for item removal. It provides flexibility and efficiency in handling user interactions and updating the displayed data.
The above is the detailed content of How to Remove and Add Items in an Android RecyclerView with a Cross Button for Deletion?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










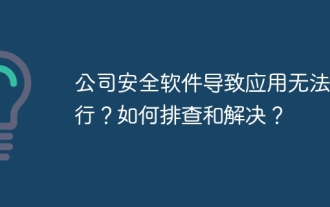
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
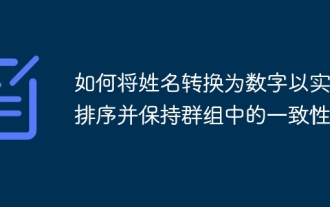
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
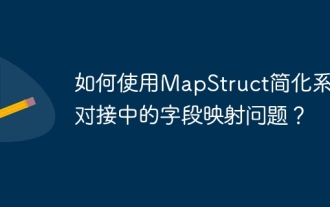
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
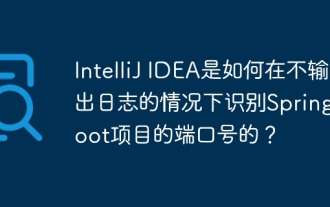
Start Spring using IntelliJIDEAUltimate version...
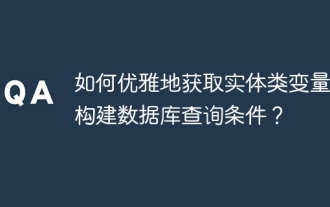
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
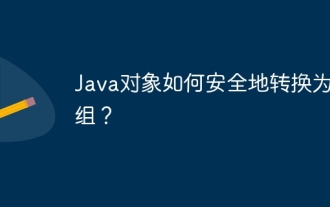
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
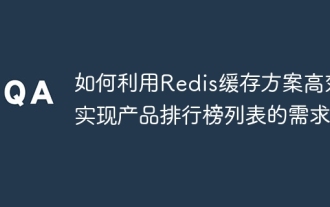
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
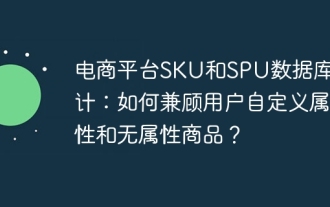
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
