How to Get the Pixel Color from a Canvas on Mousemove?
Get Pixel Color from Canvas on Mousemove
Overview
This post explores how you can retrieve the RGB values of the pixel under the mouse cursor while moving over a canvas element. We'll provide a comprehensive approach with a self-contained example.
Solution
To achieve this, first create a canvas with the desired dimensions:
<code class="html"><canvas id="example" width="200" height="60"></canvas></code>
Fill the canvas with elements such as squares:
<code class="js">const example = document.getElementById('example'); const ctx = example.getContext('2d'); ctx.fillStyle = randomColor(); ctx.fillRect(0, 0, 50, 50); ctx.fillStyle = randomColor(); ctx.fillRect(55, 0, 50, 50); ctx.fillStyle = randomColor(); ctx.fillRect(110, 0, 50, 50);</code>
Finally, add the mousemove event handler that captures the pixel values at the cursor's position:
<code class="js">$('#example').mousemove(function(e) { // Calculate the position relative to the canvas const pos = findPos(this); const x = e.pageX - pos.x; const y = e.pageY - pos.y; const coord = `x=${x}, y=${y}`; // Get the pixel value const c = this.getContext('2d'); const p = c.getImageData(x, y, 1, 1).data; // Convert to hex format const hex = "#" + ("000000" + rgbToHex(p[0], p[1], p[2])).slice(-6); // Display the color information $('#status').html(coord + "<br>" + hex); });</code>
Utility Functions
This code relies on supporting functions for finding the element's position and converting RGB values to hex. Define these functions as follows:
<code class="js">function findPos(obj) { let curleft = 0, curtop = 0; if (obj.offsetParent) { do { curleft += obj.offsetLeft; curtop += obj.offsetTop; } while (obj = obj.offsetParent); return { x: curleft, y: curtop }; } return undefined; } function rgbToHex(r, g, b) { if (r > 255 || g > 255 || b > 255) throw "Invalid color component"; return ((r << 16) | (g << 8) | b).toString(16); } function randomInt(max) { return Math.floor(Math.random() * max); } function randomColor() { return `rgb(${randomInt(256)}, ${randomInt(256)}, ${randomInt(256)})`; }</code>
Live Example
Visit the following link to see the full example in action:
https://bl.ocks.org/wayneburkett/ca41a5245a9f48766b7bc881448f9203
The above is the detailed content of How to Get the Pixel Color from a Canvas on Mousemove?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










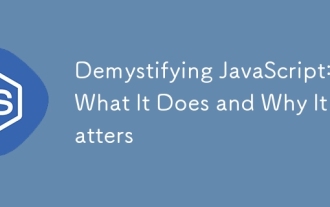
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
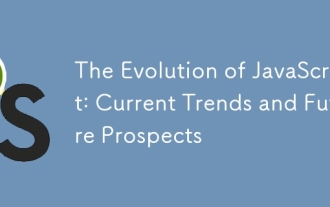
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
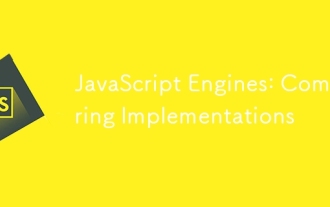
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
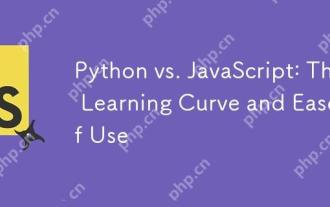
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
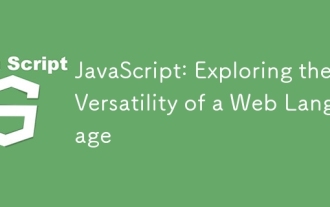
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
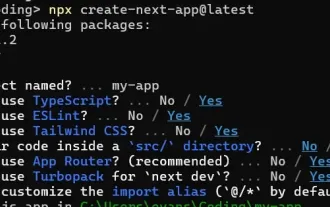
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
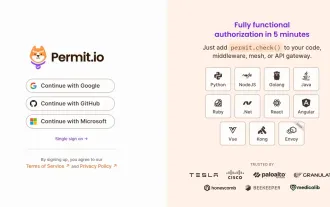
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
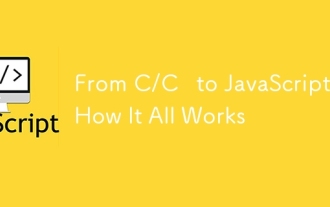
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
