


How can I retrieve a Service object by name and print its attributes using the Kubernetes Go library?
Getting Started with the Kubernetes Go Library: A Simple Client Application
When working with Kubernetes, the Go library provides a convenient interface for interacting with the API. However, documentation and examples can sometimes be out of sync with the latest version of the library. To address this, let's dive into a simple example that demonstrates how to get started.
Objective: Retrieve a Service object by name and print its attributes, such as nodePort.
Solution:
After experimenting and seeking guidance from the Kubernetes Slack channel, the following code snippet provides a working example:
<code class="go">package main import ( "fmt" "log" "github.com/kubernetes/kubernetes/pkg/api" client "github.com/kubernetes/kubernetes/pkg/client/unversioned" ) func main() { config := client.Config{ Host: "http://my-kube-api-server.me:8080", } c, err := client.New(&config) if err != nil { log.Fatalln("Can't connect to Kubernetes API:", err) } s, err := c.Services(api.NamespaceDefault).Get("some-service-name") if err != nil { log.Fatalln("Can't get service:", err) } fmt.Println("Name:", s.Name) for p, _ := range s.Spec.Ports { fmt.Println("Port:", s.Spec.Ports[p].Port) fmt.Println("NodePort:", s.Spec.Ports[p].NodePort) } }</code>
Implementation:
- Create a Config object: This specifies the host address of the Kubernetes API server.
- Create a client: The New function establishes a connection to the API server based on the provided configuration.
- Get the Service object: Use the Services and Get methods to retrieve the Service object by name from the default namespace.
- Print the attributes: Loop through the service ports and print their port and nodePort attributes.
Note: While it's possible to achieve the same result using the RESTful API, utilizing the Go library allows for more streamlined and idiomatic code.
The above is the detailed content of How can I retrieve a Service object by name and print its attributes using the Kubernetes Go library?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










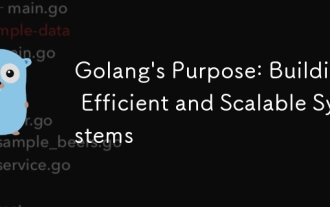
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
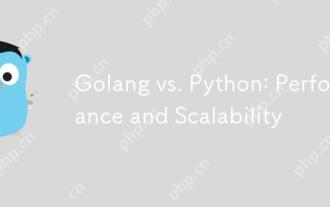
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
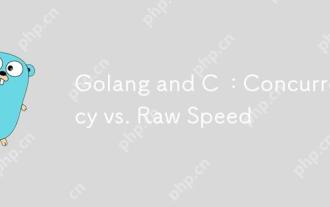
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
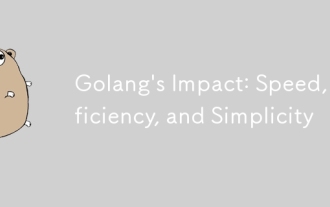
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
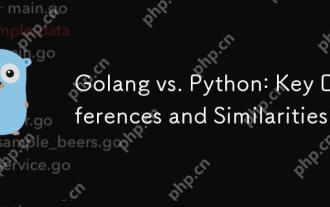
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
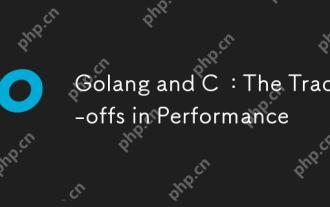
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
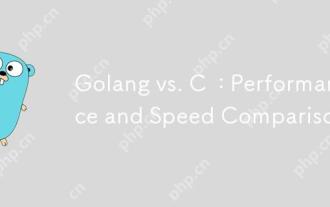
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
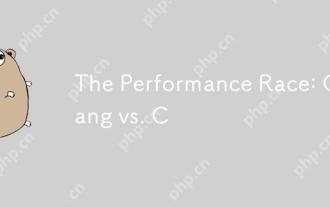
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
