


How to Extract the Complete Subject or Issuer DN from an X509 Certificate in Go?
Retrieve Complete Subject or Issuer DN from X509 Certificate in Go
Retrieving the complete subject or issuer Distinguished Name (DN) from an X509 certificate as a string can be accomplished using a few steps.
Solution Details:
-
Map OIDs to Attribute Names:
- Create a map to associate OIDs (Object Identifiers) to attribute names.
-
Convert RDNSequence to String:
- Convert the RDNSequence (Relative Distinguished Name Sequence) to a slice of strings, where each string represents an attribute (e.g., "CN=common name").
-
Build Subject DN String:
- Iterate over the slice of strings and build the subject DN string by concatenating the attribute names and values.
-
Call the Function:
- Invoke the getDNFromCert function with the certificate subject or issuer as an argument.
Example Usage:
<code class="go">func main() { // Obtain the X509 certificate x509Cert, err := LoadCert(pemBytes) if err != nil { // Handle error } // Retrieve subject DN subj, err := getDNFromCert(x509Cert.Subject, "/") if err != nil { // Handle error } fmt.Println("Subject DN:", subj) }</code>
Function Definition:
<code class="go">func getDNFromCert(namespace pkix.Name, sep string) (string, error) { subject := []string{} for _, s := range namespace.ToRDNSequence() { for _, i := range s { if v, ok := i.Value.(string); ok { subject = append(subject, fmt.Sprintf("%v=%v", i.Type.String(), v)) } else { subject = append(subject, fmt.Sprintf("%v=%v", i.Type.String(), i.Value)) } } } return sep + strings.Join(subject, sep), nil }</code>
Expected Output:
Subject DN: /C=US/O=some organization/OU=unit/CN=common name
The above is the detailed content of How to Extract the Complete Subject or Issuer DN from an X509 Certificate in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










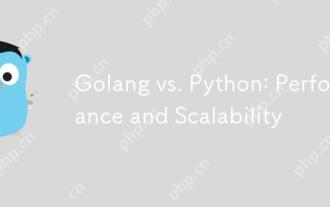
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
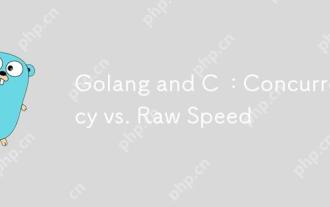
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
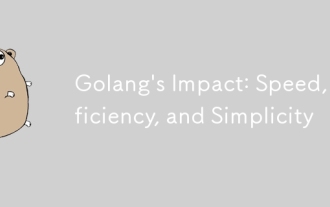
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
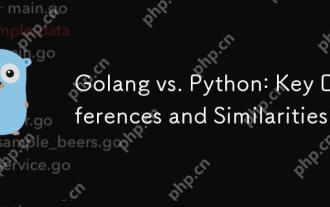
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
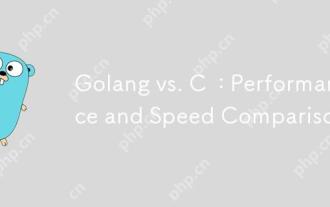
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
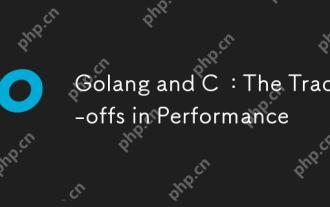
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
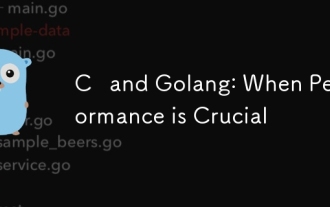
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
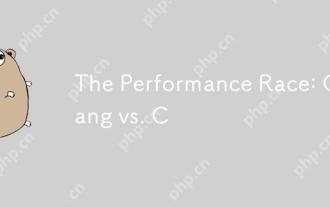
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
