How to Access Union Fields in Go with CGo: A Simplified Approach?
Converting Union Fields to Go Types in CGo
In CGo, unions are represented as byte arrays of sufficient size to hold the largest member. To access a specific union field in Go, pointer conversions are typically required.
In the given code, a value union is being accessed within a _GNetSnmpVarBind C structure. The objective is to retrieve the ui32v field, which holds an array of 32-bit unsigned integers.
Using a [8]byte array to represent the union, as in the original code, is correct. However, the conversion to a Go type that points to a C guint32 can be simplified using the address of the array.
The corrected code uses the following steps:
-
Get the address of the first element in the value union:
<code class="go">addr := &data.value[0]</code>
Copy after login -
Cast the address to a (*C.guint32) type using unsafe.Pointer:
<code class="go">cast := (**C.guint32)(unsafe.Pointer(addr))</code>
Copy after login -
Dereference the cast to obtain the value of the union field:
<code class="go">guint32_star := *cast</code>
Copy after login
Using this method, the pointer guint32_star points directly to the ui32v field of the C _GNetSnmpVarBind structure. No additional conversions are necessary to use the pointer to manipulate the array of 32-bit unsigned integers.
The above is the detailed content of How to Access Union Fields in Go with CGo: A Simplified Approach?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










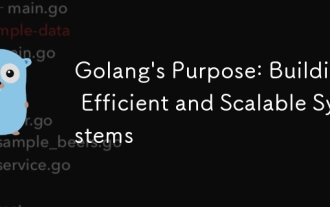
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
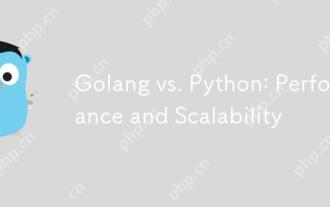
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
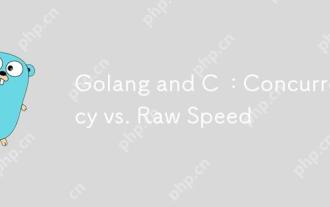
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
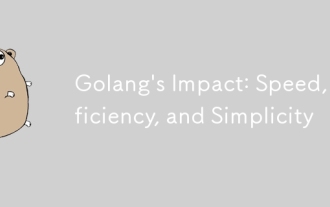
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
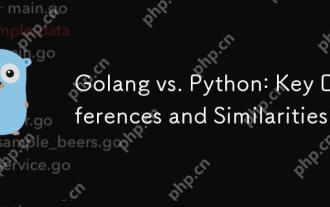
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
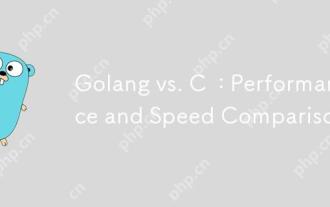
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
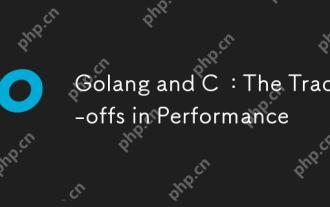
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
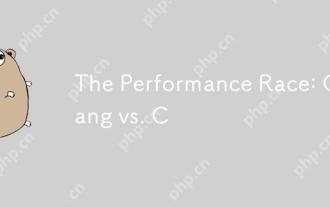
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
