


How to correctly read a PDF file from the assets folder in an Android application?
Read a PDF File from Assets Folder
Reading a PDF file from an assets folder in an Android application involves some specific steps.
In the provided code, an issue arises with the file path specified for the "abc.pdf" file. The path used, "android.resource://com.project.datastructure/assets/abc.pdf," is incorrect.
To resolve this, follow these steps:
- Copy the "abc.pdf" file to the application's internal storage: Use the CopyReadAssets() method to copy the file from the assets folder to the internal storage. This ensures that the file is accessible by the application.
- Specify the correct file path: Instead of using the assets folder path, use the file path in the application's internal storage, which would be Uri.fromFile(file), where file is the File object representing the copied PDF file.
- Set the correct permissions: Ensure that you include the WRITE_EXTERNAL_STORAGE permission in the application's AndroidManifest.xml file to allow the application to write to the internal storage.
Here's the updated code with the necessary modifications:
<code class="java">public class SampleActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); CopyReadAssets(); } private void CopyReadAssets() { AssetManager assetManager = getAssets(); InputStream in = null; OutputStream out = null; File file = new File(getFilesDir(), "abc.pdf"); try { in = assetManager.open("abc.pdf"); out = openFileOutput(file.getName(), Context.MODE_WORLD_READABLE); copyFile(in, out); in.close(); in = null; out.flush(); out.close(); out = null; } catch (Exception e) { Log.e("tag", e.getMessage()); } Intent intent = new Intent(Intent.ACTION_VIEW); intent.setDataAndType( Uri.fromFile(file), "application/pdf"); startActivity(intent); } private void copyFile(InputStream in, OutputStream out) throws IOException { byte[] buffer = new byte[1024]; int read; while ((read = in.read(buffer)) != -1) { out.write(buffer, 0, read); } } }</code>
This updated code will correctly copy the "abc.pdf" file to the application's internal storage and use the correct file path to open the PDF in a third-party PDF viewer.
The above is the detailed content of How to correctly read a PDF file from the assets folder in an Android application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










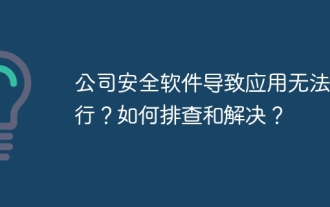
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
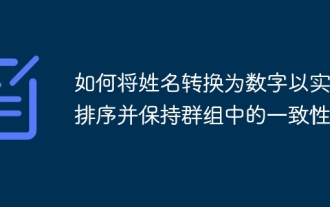
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
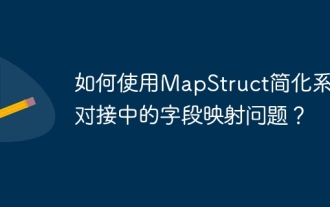
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
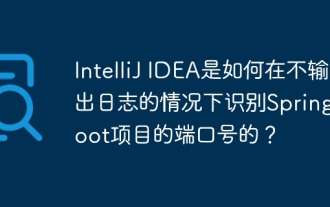
Start Spring using IntelliJIDEAUltimate version...
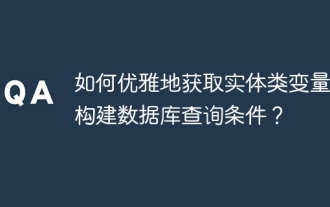
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
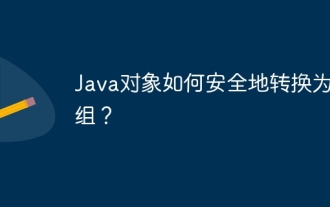
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
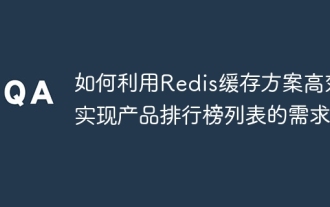
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
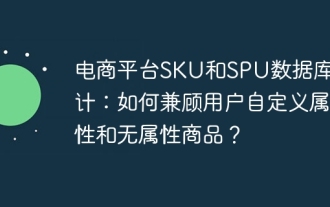
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
