


How to Resolve \'The system cannot find the path specified\' Error When Accessing Files in Java?
Resolving File Path Issues in Java When Encountering "The system cannot find the path specified"
In your Java project, you encounter an error when attempting to access a text file from a specified relative path. This error arises from the inability of the java.io.File class to locate the designated path.
To address this issue, it is recommended to retrieve the file from the classpath instead of relying on the file system. By doing so, you eliminate the need for relative paths and ensure that the file is obtained regardless of the current working directory.
Assuming that the ListStopWords.txt file resides in the same package as the FileLoader class, the following code demonstrates how to obtain the file from the classpath:
<code class="java">URL url = getClass().getResource("ListStopWords.txt"); File file = new File(url.getPath());</code>
Alternatively, if your sole purpose is to acquire an InputStream for the file, you can utilize the following:
<code class="java">InputStream input = getClass().getResourceAsStream("ListStopWords.txt");</code>
This approach is preferred as it accommodates various file system representations, including virtual file systems and network paths.
Moreover, if the file is a properties file containing key-value pairs, you can directly load it from the InputStream:
<code class="java">Properties properties = new Properties(); properties.load(getClass().getResourceAsStream("ListStopWords.txt"));</code>
Please note that if you are accessing the file from within a static context, you should replace getClass() with YourClass.class (where YourClass represents the name of your class).
The above is the detailed content of How to Resolve \'The system cannot find the path specified\' Error When Accessing Files in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










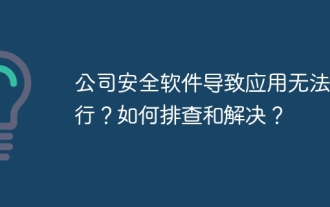
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
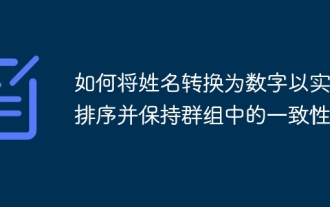
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
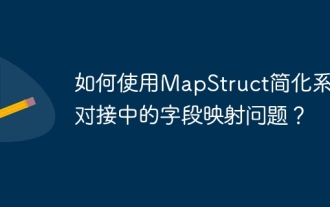
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
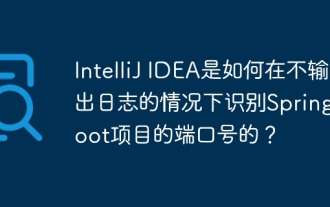
Start Spring using IntelliJIDEAUltimate version...
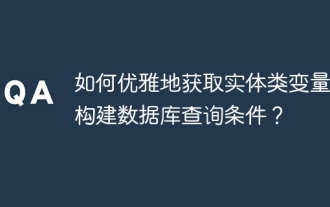
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
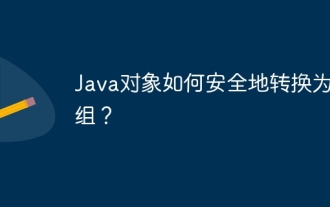
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
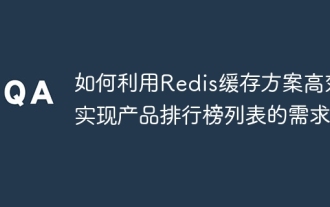
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
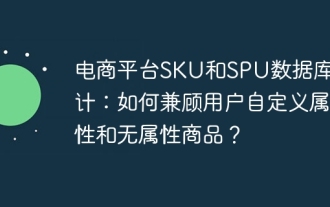
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
