


How does the defer keyword work in Go and what problem does it solve in this code example?
How the Defer Keyword Works in Go
In Go, the defer keyword is used to defer the execution of a function call until the surrounding function returns. The parameters and function value of the deferred call are evaluated as usual when the defer statement is executed, but the actual function is invoked just before the surrounding function's return statement.
Evaluation Order
The evaluation order of statements with defer keyword follows a last-in, first-out (LIFO) approach. For example, consider the following code:
<code class="go">defer func1()() defer func2()() fmt.Println("main function")</code>
In this example, the call to func1() will be deferred and executed immediately before the return of the main function. Similarly, the call to func2() will be deferred and executed before the call to func1().
Real-World Example
A common use case for defer is to automatically release resources before a function returns. For example, a file handle can be opened at the beginning of a function and closed using defer before the function returns.
Deferring a Feature Implementation
Consider the example in the problem:
<code class="go">defer fmt.Println("Your age is:", getAge(age)) // calls getAge defer fmt.Println("Your ticket price is:", printTicket(age, ticketprice)) // calls printTicket</code>
In this code, the call to getAge() is deferred before the call to printTicket(). This means that the evaluation of age and the calculation of ticketPrice will occur immediately, while the actual printing of the age and ticket price will be deferred until the function returns.
By using defer, you can ensure that the age and ticket price are always printed, even if an error occurs during the function execution.
Solution to the Problem
The original code in the problem had an issue where the printTicket function was not being called correctly for ages greater than 13. This was because the ticketPrice variable was being declared but never assigned a value. By using defer, we can ensure that the ticket price is calculated correctly before it is printed.
Here's a corrected version of the code:
<code class="go">package main import "fmt" func main() { var age int defer fmt.Println("Your age is:", getAge(&age)) defer fmt.Println("Your ticket price is:", printTicket(age)) } func printTicket(age int) float64 { var ticketPrice float64 switch { case age <= 13: ticketPrice = 9.99 case age > 13 && age < 65: ticketPrice = 19.99 case age >= 65: ticketPrice = 12.99 } return ticketPrice } func getAge(age *int) int { fmt.Println("What is your age?") fmt.Scan(age) for *age < 0 || *age > 100 { fmt.Println("That cannot be, please enter your age again") fmt.Scan(age) } return *age }</code>
With this fix, the code will correctly print the ticket price based on the user's age.
The above is the detailed content of How does the defer keyword work in Go and what problem does it solve in this code example?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










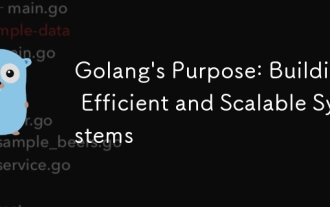
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
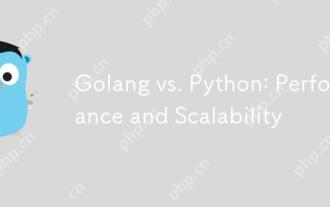
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
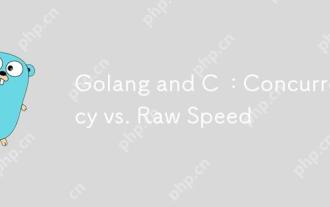
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
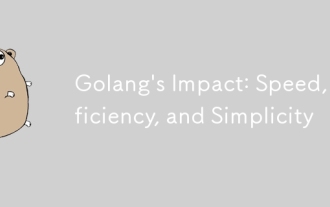
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
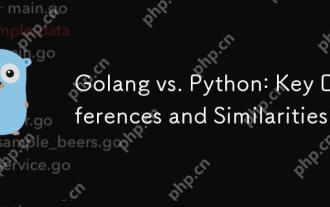
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
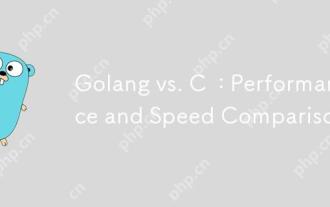
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
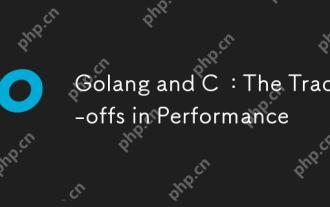
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
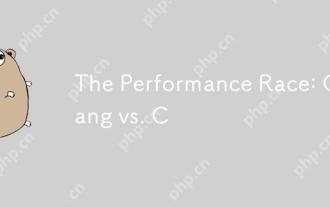
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
