


How Does `std::shared_ptr` Preserve Object Destruction Behavior Despite Type Erasure?
std::shared_ptr
The inquiry revolves around the puzzling functionality of std::shared_ptr
<code class="cpp">#include <memory> #include <iostream> #include <vector> int main() { std::cout << "At begin of main.\ncreating std::vector<std::shared_ptr<void>>" << std::endl; std::vector<std::shared_ptr<void>> v; { std::cout << "Creating test" << std::endl; v.push_back(std::shared_ptr<test>(new test())); std::cout << "Leaving scope" << std::endl; } std::cout << "Leaving main" << std::endl; return 0; }</code>
The code exhibits the following output:
At begin of main. creating std::vector<std::shared_ptr<void>> Creating test Test created Leaving scope Leaving main Test destroyed
Understanding Type Erasure
The key to this behavior lies in the type erasure performed by std::shared_ptr. When initializing a new instance, std::shared_ptr stores an internal deleter function. This function, by default, invokes the delete operator upon destruction of the shared_ptr. This mechanism ensures that the destructor of the object pointed to is invoked at the appropriate time, regardless of the type of the shared_ptr.
Consequence of Casting
Casting a std::shared_ptr
Standard Compliance and Future Implications
Regarding the guaranteed behavior of this technique, it's crucial to note that the internal implementation of std::shared_ptr may vary across different compilers and platforms. While type erasure has been a fundamental aspect of shared_ptrs, future changes to its implementation may potentially impact the described functionality.
Therefore, relying solely on this behavior is not a recommended practice and should be avoided in production code. Instead, alternative approaches, such as using std::function or a custom deleter class, are more robust and will provide consistent behavior across various implementations.
The above is the detailed content of How Does `std::shared_ptr` Preserve Object Destruction Behavior Despite Type Erasure?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


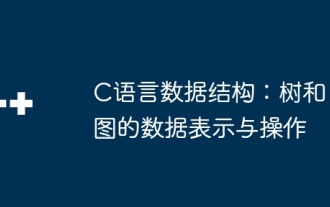
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
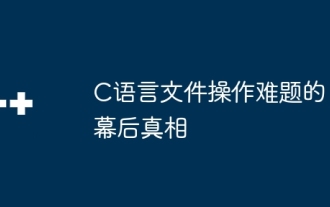
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
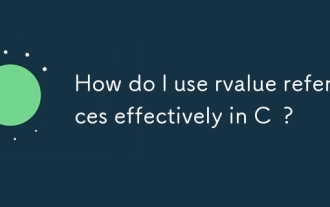
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
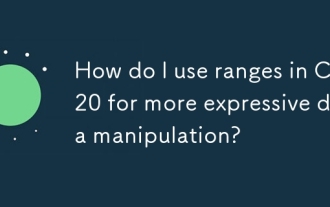
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
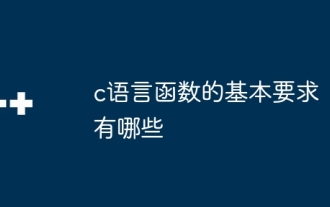
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
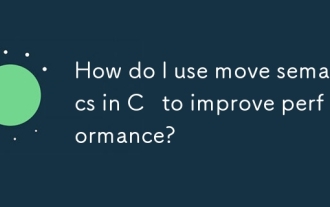
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
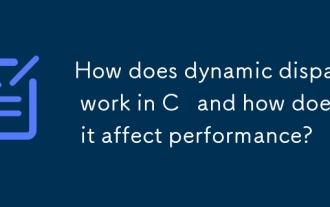
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
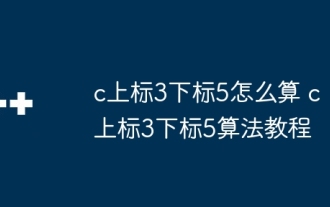
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
