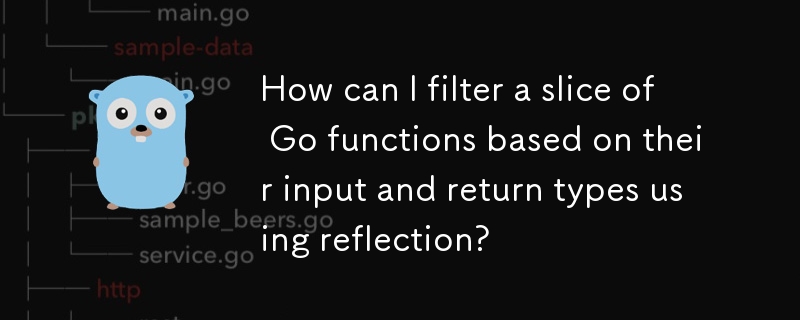
Selecting Functions with Desired Return Types or Inputs in Golang
Suppose you have an arbitrary list of functions stored in a slice or array. How can you effectively filter these functions to include only those that adhere to specific criteria, such as functions returning integers or taking integers as input?
Enter the realm of reflection in Golang, a powerful tool for introspecting and manipulating the structure of programs at runtime. Using the reflect package, we can dynamically examine the types and signatures of functions to implement the desired selection.
To demonstrate, consider the following scenario: we want to print functions from a list that either accept an integer as input or return an integer. Here's how we approach this task:
-
Create a Slice of Functions:
Start by defining a slice named "funcs" to store arbitrary functions. Each function is represented using the interface{} type to accommodate any function type.
-
Populate the Slice:
Populate the "funcs" slice with functions. The example includes three functions: one returning an integer and accepting an integer, one returning an integer and accepting a string, and one returning a string and accepting a string.
-
Iterate Over Functions:
Use a range-based loop to iterate over each function in the "funcs" slice.
-
Extract Function Metadata:
For each function, we use reflect.ValueOf to obtain its corresponding Value object. This object provides access to its Type and other metadata.
-
Inspect Function Signature:
Examine the function's type (functype) and inspect both its input and output parameters.
-
Check for Desired Types:
Iterate over the function's input and output parameters (NumIn and NumOut) and check if they match the specified condition. For instance, we check if the type string is "int" for input parameters and output parameters.
-
Print Selected Functions:
If the function meets the desired criteria, print it to the output using fmt.Println.
By following these steps, you can effectively select functions from a list based on their return types or input parameters. This code helps you identify and utilize functions that meet specific requirements, enhancing your program's flexibility and customization.
The above is the detailed content of How can I filter a slice of Go functions based on their input and return types using reflection?. For more information, please follow other related articles on the PHP Chinese website!