


How do the \'levels\', \'keys\', and \'names\' arguments in Pandas\' concat function work to create a MultiIndex?
What are the 'levels', 'keys', and names arguments for in Pandas' concat function?
1. Introduction
The pandas.concat() function is a powerful tool for combining multiple Series or DataFrames along a specified axis. It offers a number of optional arguments, including levels, keys, and names, which can be used to customize the resulting MultiIndex.
2. Levels
The levels argument is used to specify the levels of the resulting MultiIndex. By default, Pandas will infer the levels from the keys argument. However, you can override the inferred levels by passing a list of sequences to the levels argument.
For example, the following code concatenates two DataFrames along the rows, using a MultiIndex with two levels:
<code class="python">df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) df2 = pd.DataFrame({'C': [5, 6], 'D': [7, 8]}) df = pd.concat([df1, df2], keys=['df1', 'df2'], levels=['level1', 'level2']) print(df) level1 level2 A B C D 0 df1 1 1 3 5 7 1 df1 2 2 4 6 8</code>
In this example, the levels argument is a list of two sequences: ['level1', 'level2']. This creates a MultiIndex with two levels: 'level1' and 'level2'. The keys argument is a list of two strings: ['df1', 'df2']. This assigns the values 'df1' and 'df2' to the first and second levels of the MultiIndex, respectively.
3. Keys
The keys argument is used to specify the keys for the resulting MultiIndex. By default, Pandas will use the index labels of the input objects as the keys. However, you can override the default keys by passing a list of values to the keys argument.
For example, the following code concatenates two DataFrames along the rows, using a MultiIndex with three levels:
<code class="python">df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) df2 = pd.DataFrame({'C': [5, 6], 'D': [7, 8]}) df = pd.concat([df1, df2], keys=[('A', 'B'), ('C', 'D')]) print(df) level1 level2 A B C D 0 A B 1 3 5 7 1 C D 2 4 6 8</code>
In this example, the keys argument is a list of two tuples: [('A', 'B'), ('C', 'D')]. This creates a MultiIndex with three levels: 'level1', 'level2', and 'level3'. The keys argument assigns the values 'A' and 'B' to the first level of the MultiIndex, and the values 'C' and 'D' to the second level of the MultiIndex.
4. Names
The names argument is used to specify the names of the levels of the resulting MultiIndex. By default, Pandas will use the names of the index labels of the input objects as the names of the levels. However, you can override the default names by passing a list of strings to the names argument.
For example, the following code concatenates two DataFrames along the rows, using a MultiIndex with two levels:
<code class="python">df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) df2 = pd.DataFrame({'C': [5, 6], 'D': [7, 8]}) df = pd.concat([df1, df2], keys=['df1', 'df2'], names=['level1', 'level2']) print(df) level1 level2 A B C D 0 df1 1 1 3 5 7 1 df1 2 2 4 6 8</code>
In this example, the names argument is a list of two strings: ['level1', 'level2']. This assigns the names 'level1' and 'level2' to the first and second levels of the MultiIndex, respectively.
The above is the detailed content of How do the \'levels\', \'keys\', and \'names\' arguments in Pandas\' concat function work to create a MultiIndex?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










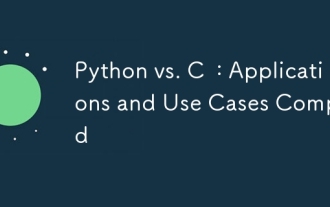
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
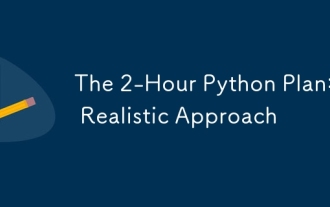
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
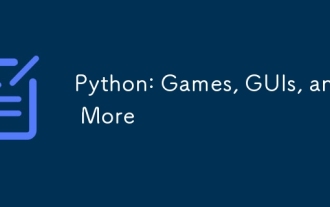
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
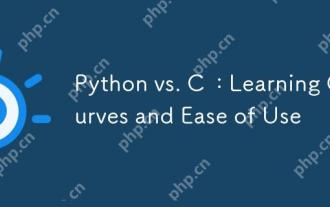
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
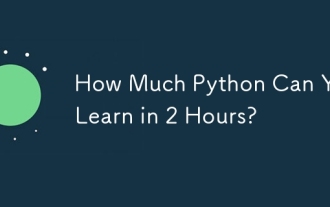
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
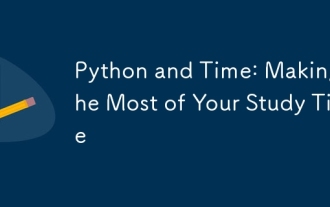
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
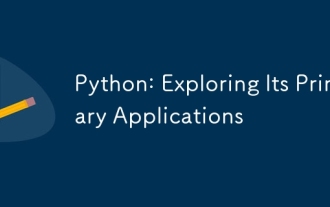
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
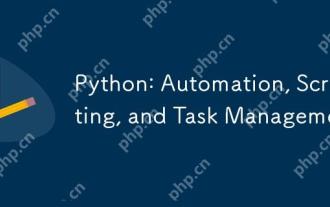
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
