How to force IPv4 or IPv6 connections in Go\'s net/http Client?
Oct 31, 2024 pm 08:48 PMForcing IPv4 or IPv6 in Go's net/http Client
In Go, the net/http package provides a versatile HTTP client for making requests. It offers flexibility in customizing the behavior of the client through its Transport field. This article will explore how to force the client to use either IPv4 or IPv6 when establishing connections.
Detecting IPv6-Only Domains
To determine if a domain is IPv6-only, we can create a custom DialContext function that checks the network parameter passed to the Control method. If the network is "tcp4," this indicates an IPv4-only connection, and we can cancel the connection.
<code class="go">func ModifiedTransport() { var MyTransport = &amp;http.Transport{ DialContext: (&amp;net.Dialer{ DualStack: false, Control: func(network, address string, c syscall.RawConn) error { if network == "tcp4" { return errors.New("you should not use ipv4") } return nil }, }).DialContext, MaxIdleConns: 100, IdleConnTimeout: 90 * time.Second, TLSHandshakeTimeout: 10 * time.Second, ExpectContinueTimeout: 1 * time.Second, } var myClient = http.Client{Transport: MyTransport} resp, myerr := myClient.Get("http://www.github.com") if myerr != nil { fmt.Println("request error") return } }</code>
Forcing IPv6 Connections
To force an IPv6 connection, we can specify "tcp6" as the network parameter in the Control function:
<code class="go">func ModifiedTransport() { var MyTransport = &amp;http.Transport{ DialContext: (&amp;net.Dialer{ DualStack: false, Control: func(network, address string, c syscall.RawConn) error { if network != "tcp6" { return errors.New("you should use ipv6") } return nil }, }).DialContext, MaxIdleConns: 100, IdleConnTimeout: 90 * time.Second, TLSHandshakeTimeout: 10 * time.Second, ExpectContinueTimeout: 1 * time.Second, } var myClient = http.Client{Transport: MyTransport} }</code>
By controlling the network parameter in the Control function, we can enforce IPv4 or IPv6 connectivity as desired. This approach provides flexibility in customizing the behavior of Go's net/http client for specific networking requirements.
The above is the detailed content of How to force IPv4 or IPv6 connections in Go\'s net/http Client?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
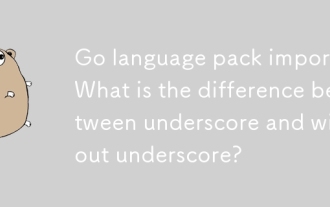
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
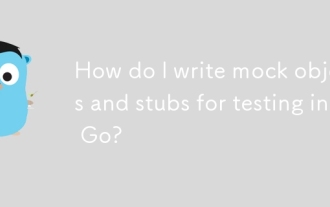
How do I write mock objects and stubs for testing in Go?
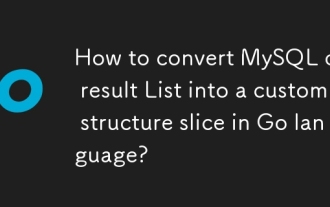
How to convert MySQL query result List into a custom structure slice in Go language?
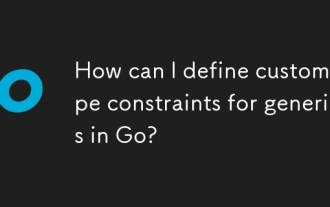
How can I define custom type constraints for generics in Go?
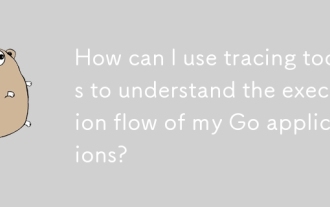
How can I use tracing tools to understand the execution flow of my Go applications?
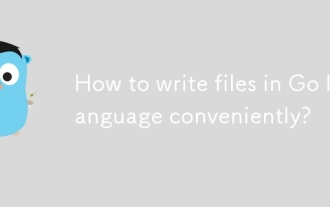
How to write files in Go language conveniently?
