Can I Join Tables in MySQL Based on Values Separated by Semicolons?
Can I Resolve This with Pure MySQL? (Joining on ';' Separated Values in a Column)
Question:
I have data in multiple tables that I need to join based on ';' (semicolon) separated values in a column. I cannot use PHP or any other language to manipulate the results. Is there a way to achieve this using only MySQL?
Answer:
Yes, it is possible to join on ';' separated values in a column using pure MySQL. Below is a detailed explanation of the solution:
Converting the Delimited String into Rows
The key is to convert the ';' delimited string into a series of rows. This can be achieved using the following steps:
- Create a table named integerseries containing the numbers from 1 to the maximum number of items in the delimited string.
- Use the COUNT_IN_SET and VALUE_IN_SET functions to count the number of items in the delimited string and extract each item based on its position.
- Join the user_resource table with the integerseries table using a cross join. This will generate rows for each item in the delimited string.
Query to Normalize the User_resource Table
<code class="sql">SELECT user_resource.user, user_resource.resources, COUNT_IN_SET(user_resource.resources, ';') AS resources_count, isequence.id AS resources_index, VALUE_IN_SET(user_resource.resources, ';', isequence.id) AS resources_value FROM user_resource JOIN integerseries AS isequence ON isequence.id <= COUNT_IN_SET(user_resource.resources, ';') ORDER BY user_resource.user, isequence.id</code>
Joining the Normalized Table with the Resource Table
Once the user_resource table is normalized, it can be joined with the resource table to obtain the desired result.
<code class="sql">SELECT user_resource.user, resource.data FROM user_resource JOIN integerseries AS isequence ON isequence.id <= COUNT_IN_SET(user_resource.resources, ';') JOIN resource ON resource.id = VALUE_IN_SET(user_resource.resources, ';', isequence.id) ORDER BY user_resource.user, resource.data</code>
Sample Input and Output
user_resource Table:
user | resources |
---|---|
user1 | 1;2;4 |
user2 | 2 |
user3 | 3;4 |
resource Table:
id | data |
---|---|
1 | data1 |
2 | data2 |
3 | data3 |
4 | data4 |
5 | data5 |
Normalizeduser_resource Table:
user | resources | resources_count | resources_index | resources_value |
---|---|---|---|---|
user1 | 1;2;4 | 3 | 1 | 1 |
user1 | 1;2;4 | 3 | 2 | 2 |
user1 | 1;2;4 | 3 | 3 | 4 |
user2 | 2 | 1 | 1 | 2 |
user3 | 3;4 | 2 | 1 | 3 |
user3 | 3;4 | 2 | 2 | 4 |
Output (Joined Result):
user | data |
---|---|
user1 | data1 |
user1 | data2 |
user1 | data4 |
user2 | data2 |
The above is the detailed content of Can I Join Tables in MySQL Based on Values Separated by Semicolons?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
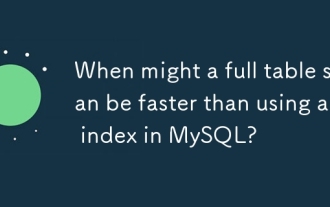
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
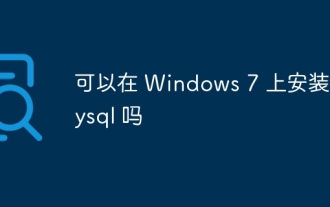
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
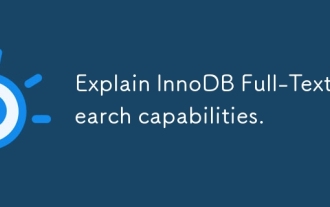
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
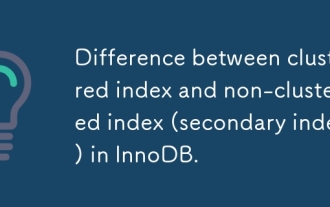
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
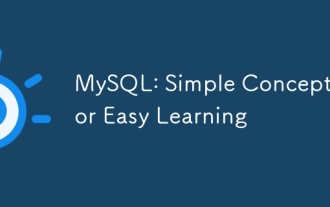
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
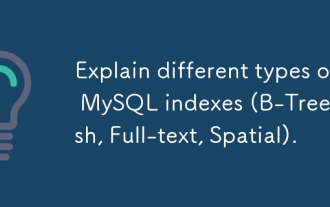
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
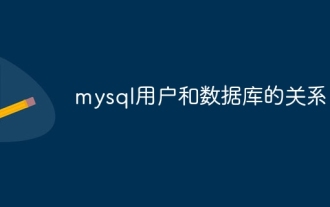
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
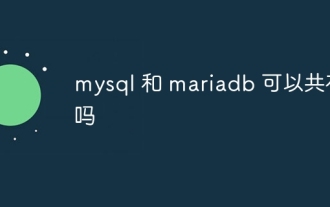
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
