Basic Golang - Equality Comparison
This post is part of a series where I intend to share the essential basics for developing with GoLang.
Logical comparisons using the == or != operators don't have much mystery. Or your code compares whether the values are the same or different.
But there are some important details to know about what you're comparing.
Comparable Interface
The first thing is to know what can be compared with these operators, the most obvious place to know this is the Go documentation: section on comparators
And another option is to look at the comparable interface, which was added along with Go's generics implementation, all types that implement this interface are comparable.
So basically all primitive types defined by Go are comparable, such as: string, numbers (int, float, complex), bool.
Comparison of complex types
Some types have conditions to be comparable or not. Which is the case of: struct, slices and channels.
They are only comparable if their elements are also comparable.
The interesting thing is that Go validates this at the compilation level, helping you avoid runtime errors, for example:
//Esse código compila e a comparação funciona: func main() { test1 := struct { name string }{} test2 := struct { name string }{} if test1 == test2 { fmt.Println("Funciona") } }
In the code above I am creating two structs with equivalent attributes and the comparison works.
//Esse código não compila func main() { test1 := struct { name string attributes map[string]string }{} test2 := struct { name string attributes map[string]string }{} if test1 == test2 { fmt.Println("Cade?") } }
This code will fail to compile with the error invalid operation.
This happens because map is not a comparable type in Go.
Details about structs and map
It is very convenient to be able to compare structs with ==, since these are the types where we customize our modeling.
But even when using a non-comparable type there are some ways to simplify the code, and knowing this will save you from writing really boring conditions.
Well, let's imagine that we are working in a school system and we have the following non-comparable struct:
type student struct { ID int name string age int course string attributes map[string]string }
The good part, this struct has an ID field, which makes it a lot easier if you are working with persisted data.
Now just imagine if you are working with data that is still transient, for example if you are reading a file and need to do some type of processing before persisting this data.
You will always have the option to compare field by field, it's not convenient at all, but it works. But you can make use of struct composition as follows:
func main() { type identity struct { name string age int course string } type student struct { ID int identity attributes map[string]string } s1 := student{ identity: identity{ name: "Chuck", age: 10, course: "golang", }, attributes: map[string]string{ "last_score": "10", }, } s2 := student{ identity: identity{ name: "Chuck", age: 10, course: "golang", }, attributes: map[string]string{ "last_score": "20", }, } s3 := student{ identity: identity{ name: "Chuck", age: 12, course: "golang", }, attributes: map[string]string{ "last_score": "20", }, } if s1.identity == s2.identity { fmt.Println("Achou", s1) } if s1.identity != s3.identity { fmt.Println("Não achou") } }
The alternative to this code would be to create conditions like these:
if s1.name == s2.name && s1.age == s2.age && s1.course == s2.course { fmt.Println("Achou", s1) }
You could extract it to a function, to make it less confusing, but you would still have to maintain these comparisons.
Make good use of composition this can greatly simplify your code!
Summary
- Learn about the comparable interface
- Primitive types are comparable
- Complex types may or may not be comparable
- Use composition to your advantage.
The above is the detailed content of Basic Golang - Equality Comparison. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










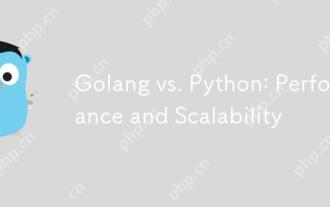
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
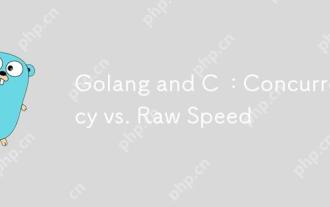
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
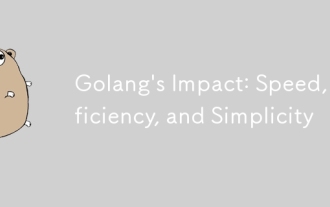
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
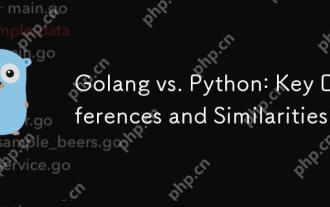
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
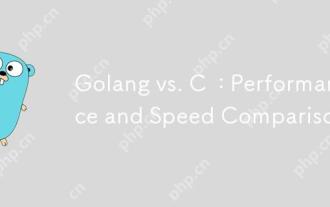
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
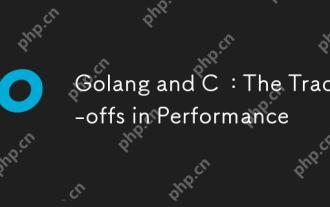
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
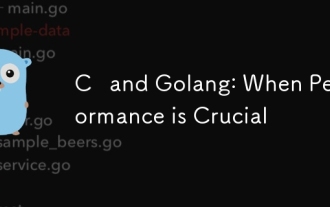
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
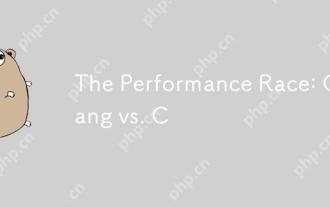
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
