How to Efficiently Reverse Streams in Java 8?
Exploring Stream Reversal Techniques in Java 8
When dealing with streams in Java 8, encountering the need to reverse their order is not uncommon. To ascertain the correct approach for reversing any stream, we delve into the specific challenge of reversing an IntStream while exploring additional solutions for reversing streams of any type.
IntStream Reversal:*
IntStream offers a range() method for generating integers within a specified range. To reverse the range, simply adjust the arguments:
<code class="java">IntStream.range(-range, 0)</code>
However, this approach is impractical, and Integer::compare cannot be used due to compiler errors. Instead, consider the following code:
<code class="java">static IntStream revRange(int from, int to) { return IntStream.range(from, to) .map(i -> to - i + from - 1); }</code>
This method reverses the range without boxing or sorting.
General Stream Reversal:
There are multiple ways to reverse streams of any type, but both require storing the elements.
Array Based Reversal:
This method uses an array to store elements for subsequent reading in reverse order:
<code class="java">@SuppressWarnings("unchecked") static <T> Stream<T> reverse(Stream<T> input) { Object[] temp = input.toArray(); return (Stream<T>) IntStream.range(0, temp.length) .mapToObj(i -> temp[temp.length - i - 1]); }</code>
Collector Based Reversal:
Collectors can be used to accumulate elements into a reversed list:
<code class="java">Stream<T> input = ... ; List<T> output = input.collect(ArrayList::new, (list, e) -> list.add(0, e), (list1, list2) -> list1.addAll(0, list2));</code>
While this is effective, it triggers many ArrayList.add(0, ...) insertions, resulting in excessive copying.
Efficient Collector Based Reversal:
To mitigate the drawback of copying, consider using an ArrayDeque, which supports efficient insertions at the front:
<code class="java">Deque<String> output = input.collect(Collector.of( ArrayDeque::new, (deq, t) -> deq.addFirst(t), (d1, d2) -> { d2.addAll(d1); return d2; }));</code>
This updated code maintains the efficiency of the Collector based reversal while eliminating unnecessary copying.
The above is the detailed content of How to Efficiently Reverse Streams in Java 8?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










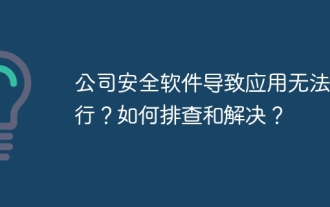
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
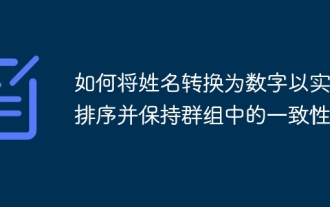
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
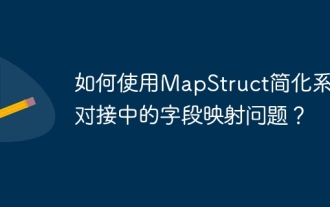
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
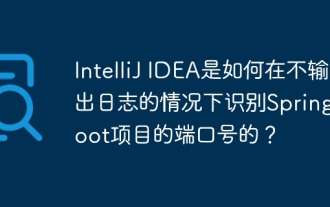
Start Spring using IntelliJIDEAUltimate version...
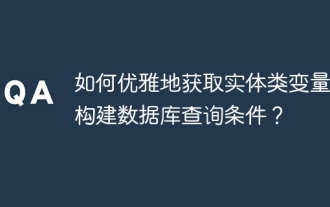
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
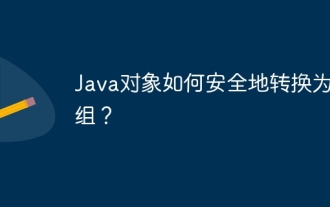
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
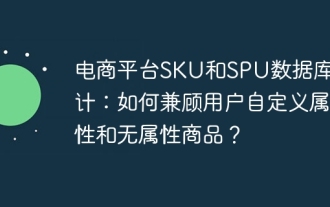
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
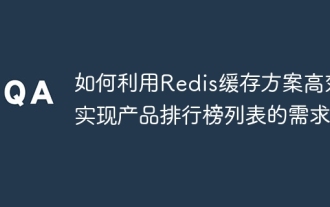
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
