


How can I dynamically update handlers in Go\'s HTTP mux without restarting the server?
Dynamically Updating Handlers in Go's HTTP Mux
In web applications, it's often desirable to modify or replace routes without having to restart the server. While there's no built-in support for this in Go's http.NewServeMux or Gorilla's mux.Router, it's possible to implement this using custom structures.
Custom Handler Structure
The solution involves creating a custom Handler structure that encapsulates both the handler function and an Enabled flag:
<code class="go">type Handler struct { http.HandlerFunc Enabled bool }</code>
Handler Map
A map of handlers is used to store the custom handlers associated with different route patterns:
<code class="go">type Handlers map[string]*Handler</code>
HTTP Handler
A custom HTTP Handler is implemented to check if a handler is enabled for the given URL path:
<code class="go">func (h Handlers) ServeHTTP(w http.ResponseWriter, r *http.Request) { path := r.URL.Path if handler, ok := h[path]; ok && handler.Enabled { handler.ServeHTTP(w, r) } else { http.Error(w, "Not Found", http.StatusNotFound) } }</code>
Integrating with Mux
The custom Handlers can be integrated with http.NewServeMux or mux.Router by implementing the HandleFunc method:
<code class="go">func (h Handlers) HandleFunc(mux HasHandleFunc, pattern string, handler http.HandlerFunc) { h[pattern] = &Handler{handler, true} mux.HandleFunc(pattern, h.ServeHTTP) }</code>
Example
Here's an example that dynamically disables a handler after the first request:
<code class="go">package main import ( "fmt" "net/http" ) func main() { mux := http.NewServeMux() handlers := Handlers{} handlers.HandleFunc(mux, "/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Welcome!") handlers["/"].Enabled = false }) http.Handle("/", mux) http.ListenAndServe(":9020", nil) }</code>
Conclusion
Using custom handlers, it's possible to dynamically enable and disable routes in Go's HTTP mux without restarting the server. This provides greater flexibility and control over application behavior and can streamline development and maintenance tasks.
The above is the detailed content of How can I dynamically update handlers in Go\'s HTTP mux without restarting the server?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










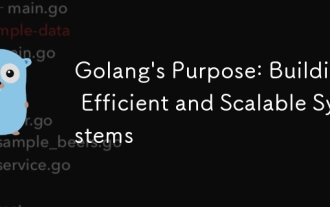
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
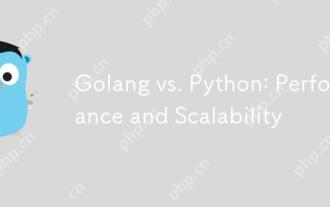
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
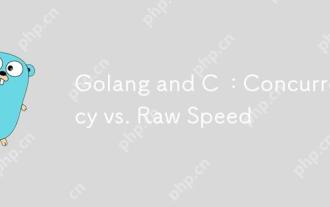
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
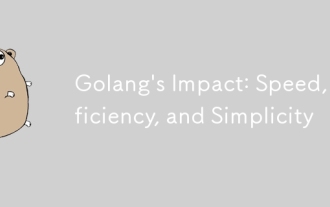
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
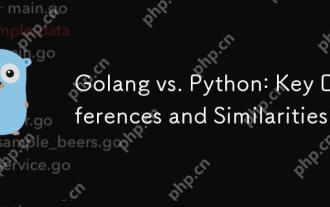
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
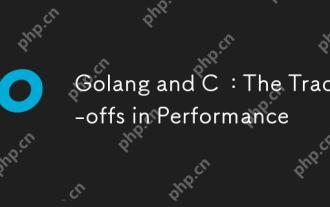
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
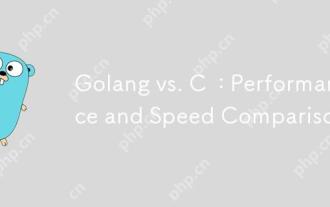
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
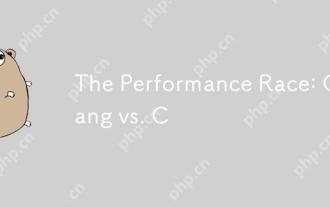
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
