How to Ensure Only One Instance of a C/C Application Runs at a Time?
Nov 01, 2024 am 05:28 AMCreating Single Instance Applications with C or C
To ensure that only one instance of an application runs concurrently, there are various techniques to consider. Here are some options and their advantages:
File Lock:
One approach is using file locking. A unique file is created by the application, and when it executes, it acquires an exclusive lock on this file. If another instance attempts to run, it will fail to acquire the lock, indicating that an instance is already running.
Mutex:
Mutexes are synchronization objects that allow multiple threads or processes to safely access shared resources without conflicts. In this case, a mutex can be used to control access to the running application. When the first instance acquires the mutex, any subsequent instances will be blocked until the mutex is released.
Unix Domain Socket:
Creating and binding a Unix domain socket with a unique name is another method. The first instance of the application successfully binds to the socket. When another instance tries to bind to the same name, it will fail and can connect to the existing socket to communicate with the first instance.
Implementation Example:
Here's an example implementation using file locking:
<code class="c">#include <sys/file.h> #include <errno.h> int main() { int pid_file = open("/var/run/my_app.pid", O_CREAT | O_RDWR, 0666); int rc = flock(pid_file, LOCK_EX | LOCK_NB); if (rc) { if (EWOULDBLOCK == errno) { // Another instance is running return 1; } } else { // This is the first instance } // Perform application logic return 0; }</code>
This approach ensures that only one instance of the application is running and has the advantage of being able to handle stale pid files.
The above is the detailed content of How to Ensure Only One Instance of a C/C Application Runs at a Time?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
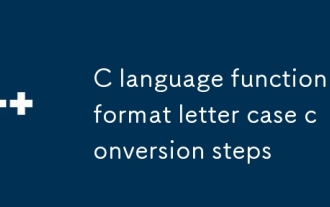
C language function format letter case conversion steps
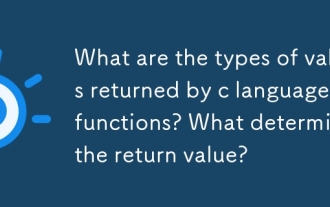
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
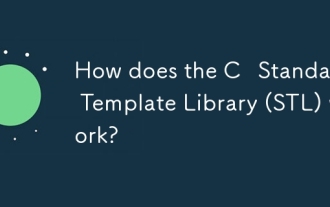
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
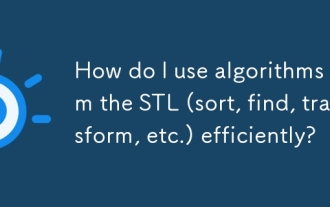
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
